A Random Password Generator is an application used to generate random passwords that help to pick a strong password for authentication to enhance security and decrease the chance of hacking.
Creating a Random Password Generator in NodeJS
This tutorial provides a complete guide to building the Random Password Generator using NodeJS.
Project Setup
Create a project folder then locate the folder in the terminal and execute the below command to initiate NPM.
npm init -y
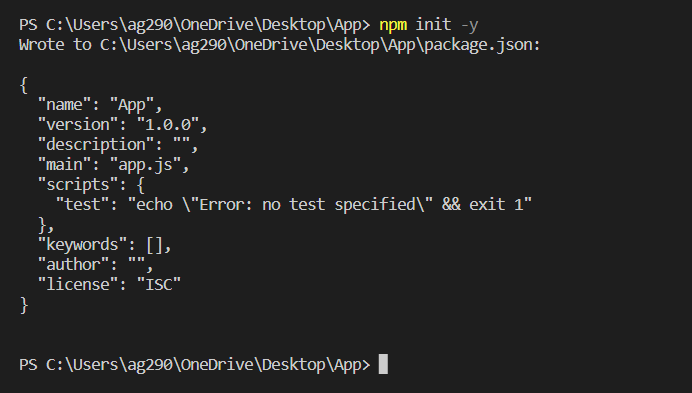
NPM stands for Node Package Manager used to install different NodeJS packages.
Express Module
For this tutorial, we will use Express which makes it easy for beginners to write NodeJS code.
Execute the below command in the terminal to install Express inside the project folder.
npm i express
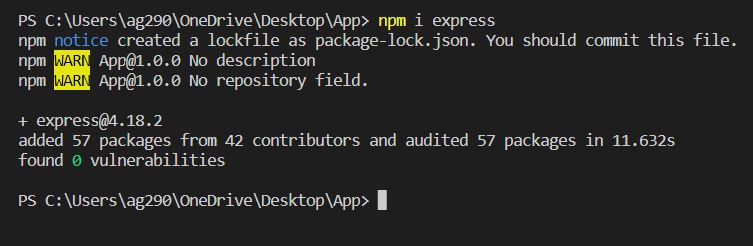
Project Structure
The project contains a JavaScript file “app.js”, where we will write code for generating random passwords, a package.json and package-lock.json file which contains the information regarding the modules installed in our project, and a node_modules folder which contains the actual module installed inside our projects.
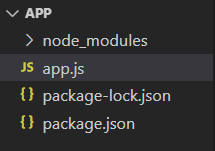
Creating Server
For using the application in the browser it is required to set up a server. A server is set up in minutes using the Express module which we have already installed in the project folder.
Below is the code for creating a server local server.
const express = require('express');
const app = express();
app.listen(3000);
Here we have imported the express module to use it inside the “app.js” file then create a constant “app” as an instance of express to use its methods. Then use listen() method that takes a PORT as an argument on which it runs the server.
Creating Functionalities
We want to generate and send a random password when the user requests the application’s home page. For this, we have to create a home route, represent “/” which is the application’s default page, and then define the functionality to generate a random password and send that password as a response when the application’s home page opens on the browser.
Below is the code for generating a random password and sending the generated password as a response to the browser.
app.get('/', (req, res) => {
var length = 12,
charset =
"@#$&*0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ@#$&*0123456789abcdefghijklmnopqrstuvwxyz",
password = "";
for (var i = 0, n = charset.length; i < length; ++i) {
password += charset.charAt(Math.floor(Math.random() * n));
}
res.send(password);
});
Here, we use the get() method to create a home route(“/”), then use a comma and pass a callback function where we have written the code to generate a random password.
For generating the password, we created a string containing letters, numbers, and characters, then use the Math.floor() and Math.random() function to get 12-digit characters from that string in the random order.
Then use the response object to send the response. This response object has a method send() which can take a string as an argument to send as a response, so we have passed the generated password as an argument to this method to send the generate random password as a response to the browser.
Complete Code for Random Password Generator in NodeJS
const express = require('express');
const app = express();
app.get('/', (req, res) => {
var length = 12,
charset =
"@#$&*0123456789ABCDEFGHIJKLMNOPQRSTUVWXYZ@#$&*0123456789abcdefghijklmnopqrstuvwxyz",
password = "";
for (var i = 0, n = charset.length; i < length; ++i) {
password += charset.charAt(Math.floor(Math.random() * n));
}
res.send(password);
});
app.listen(3000);
Run the Application
The application code is written in a single file “app.js” that runs on executing the below command in the terminal.
node app.js
Open the Application
Enter the below URL inside the search bar of a web browser to open the application.
http://localhost:3000/
Output
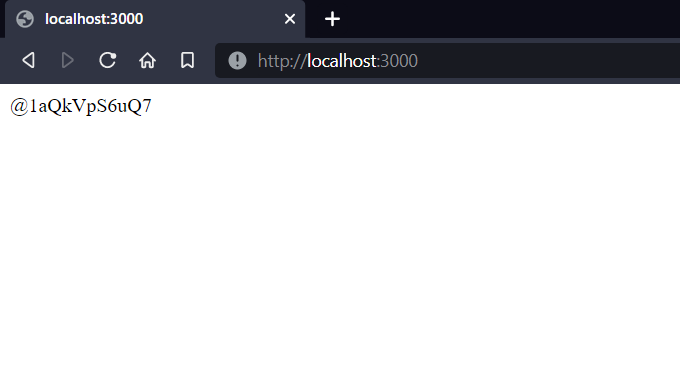
Refresh the page to generate new passwords.
Summary
A Random Password Generator is created by selecting the different combinations of letters, numbers, and characters in random order. This order should be highly randomized so that the application never generates the same password twice. Hope this tutorial guide you to create a Random Password Generator in NodeJS.
Reference
https://www.npmjs.com/package/express