MongoDB database is used to store a large number of records, these records are called documents. These documents take some space in the memory, and to free up the space occupied by irrelevant documents they can be directly removed. Document removal also helps clean irrelevant, useless, or temporary data.
Methods for Removing MongoDB Documents
Documents are removed from a MongoDB collection using the deleteOne() and deleteMany() methods.
In many applications, repositories or tutorials, you notice that the remove() method is used to remove the documents, since it is deprecated we suggested using deleteOne() and deleteMany() methods instead.
Example: deleteOne() Method
This method deletes a single document from a collection. If there are many documents then it deletes the first one.
This method can take an object and a callback function as an argument. Inside the object, the MongoDB query can be passed to select the documents. We have covered Query in another tutorial NodeJS MongoDB Query if you want to read it. Inside the callback, it returns the error if occurs or the result object.
Syntax:
database.collection(collectionName).deleteOne(query, function(err, result) {
if (err) throw err;
console.log(result);
});
where,
- database is a database object,
- collectionName is the name of a collection
- query is a query object
Example
We have multiple documents in a collection.
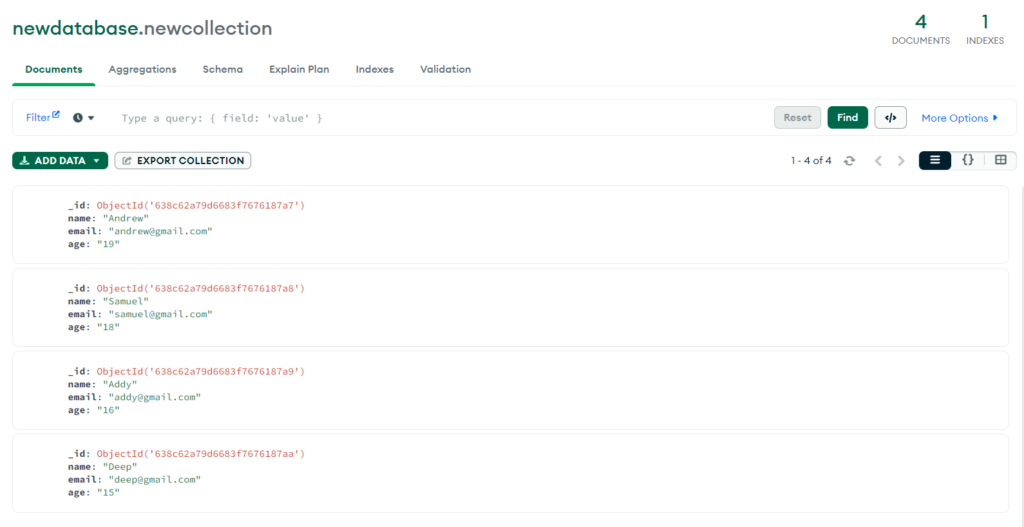
If you want to learn to insert multiple records in a collection then read our previous tutorial NodeJS MongoDB Insert Record.
Below is an example of deleting a single document from a collection.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const query = { name: "Samuel" };
database.collection("newcollection").deleteOne(query, function(err, result) {
if (err) throw err;
console.log(result);
});
});
Here the query object { name: “Samuel” } specify to delete only that document where the name field is equal to “Samuel”.
Run the Code:
To run the above code, create a project folder, and copy and paste the above code into a JavaScript file.
Then locate the folder in the terminal and execute the below command to install the MongoDB module.
npm install mongodb
Then execute the below command to run the code.
node fileName
where fileName is the name of the JavaScript file where you have inserted the code.
Output:

Example: deleteMany() Method
This method deletes multiple documents. It can also delete all the documents from a collection.
This method takes a query object as an argument used to filter the documents to delete if the query is not passed then delete all the documents from a collection.
Syntax:
database.collection(collectionName).deleteMany(query, function(err, result) {
if (err) throw err;
console.log(result);
});
where,
- database is a database object,
- collectionName is the name of a collection
- query is a query object
Example
Below is an example of deleting the documents where the name field starts with the letter “A”.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
const query = { name: /^A/ };
database.collection("newcollection").deleteMany(query, function(err, result) {
if (err) throw err;
console.log(result);
});
});
Run the Code:
To run the above code, create a project folder, and copy and paste the above code into a JavaScript file.
Then locate the folder in the terminal and execute the below command to install the MongoDB module.
npm install mongodb
Then execute the below command to run the code.
node fileName
where fileName is the name of the JavaScript file where you have inserted the code.
Output:

Verifying the Document Removal
To verify that the documents are deleted successfully, run the below code which returns the remains documents in the console.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
database.collection("newcollection").find({}).toArray(function(err, documentsArray) {
if (err) throw err;
console.log(documentsArray);
});
});
Output:
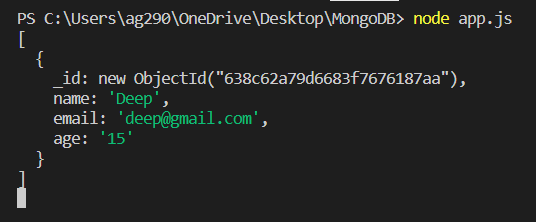
The output doesn’t have any documents where the name is equal to “Samuel” or starts with the letter “A” which verifies that the documents are successfully deleted.
Summary
MongoDB documents can be removed directly from NodeJS using the methods deleteOne() and deleteMany() taking a query to get the documents to delete from a particular collection. In the meantime, we can also get the details regarding the number of documents deleted inside the callback of these methods. Hope this tutorial helps you to understand the methods of removing MongoDB documents from a collection.
Reference
https://www.mongodb.com/docs/drivers/node/current/fundamentals/crud/write-operations/delete/