What is programming without variables? Variables form an integral part of programming by taking different forms of data as required during the execution of the program. These different forms of data are called data types & this article shall focus on exploring in detail the different data types that are available in Python.
Python Data Types
Unlike most programming languages, Python is an interpreter-based language & thereby it does not require the coder to explicitly declare the type of data that is to be assigned to the variable, it just interprets. However, in some cases, one might need to explicitly mention the type of data that is to be used so as to ensure that incorrect or invalid entries are refrained from being recorded against a particular variable.
Given below is the list of the data types available in Python:
- Numeric
- String
- Sequence
- Sets
- Boolean
- Dictionary
Python Numeric Data type
Used to denote the type of numerals assigned to any variable, numbers are further classified into the following three types.
- int – for whole numbers such as 20, 58, 3984
- float – for decimals such as 3.58, 8.90, 589.09
- complex – for denoting complex numbers such as 1+3i, 4-20i
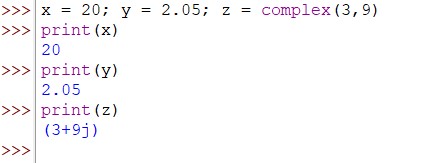
Python String Data Type
All textual data is used in Python programming with this data type. It is to be noted that all string type data is to be entered between double quotes as shown below.
a1 = str(input("Enter ID:"))
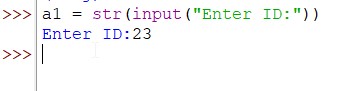
Python Sequence Data Type
This data type is used to deal with a collection of data, be it text or numbers and is in turn classified into the following:
- List – used to collate both data & text in which the order of elements can be changed.
- Tuple – used to collate data in the order of the elements which cannot be altered.
- Range – used to declare an incremental series of numbers.
list = [20, "Xenon", 20.5]
tuple = (2.79, 8.43, 0.09)
rng = range(0, 10, 2)
Python Set Data Type
A collection of unordered data is what sets stand for in Mathematics & this data type is its equivalent in Python.
When the entities in a set cannot be modified, it falls under a special category known as frozen set. In other words, frozen sets are immutable.
ar1 = (24, 89, 87, 345)
frzst = frozenset(ar1)
print(frzst)
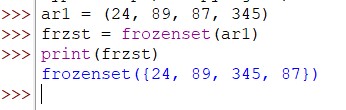
In the above image, if ‘ar1’ is left alone without being put through the forzenset( ) function, it qualifies to be a conventional set.
Python Boolean Data Type
Boolean data are obtained in Python programming through the bool( ) function which returns either True or False as a result.
x = 29
bool(x<30)
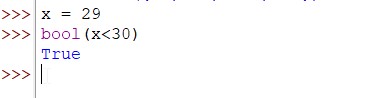
Python Dictionary Data Type
A collection of data in terms of keys & their corresponding values is called a Dictionary in Python. The following example explains it better.
dct = {'Name':['Zeb', 'Fur', 'Tik'], 'Age': [21, 8, 43]}
In the above code, ‘Name’ is the key and those that follow within the square brackets are the values for that key. The same holds good for ‘Age’ too. It is also to be noted that all pairs of keys & values are to be included within the curly braces.
How to Find the Data Type in Python?
In order to know which type of data, a particular variable uses, one can deploy the type( ) function which returns the data type that has been assigned to that variable as shown below.
x = 20; y = 2.05; z = complex(3,7)
print(type(x))
print(type(y))
print(type(z))
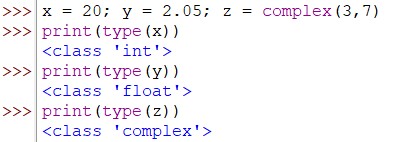
a = 'Alpha'; l = [20, "Xenon", 20.5]; ar1 = (24, 89, 87, 345)
print(type(a))
print(type(l))
print(type(ar1))
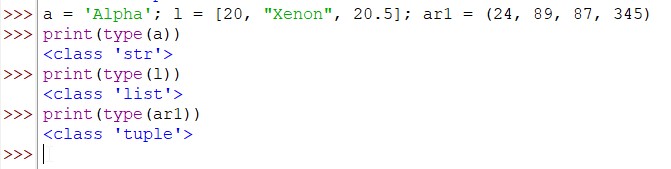
Conclusion
Now that we have reached the end of this article, hope it has elaborated on the different data types that exist in Python programming. Here’s another article that can be your definite guide to divide in Python programming. There are numerous other enjoyable & equally informative articles in CodeforGeek that might be of great help to those who are looking to level up in Python. Whilst you enjoy those, adios!
Reference
https://docs.python.org/3/library/datatypes.html