In Node.js we can easily make up a login system. We only have to send a form to the user to enter username and password, handle that form data in the server file and save that into a database. Then when the user asks for login, we just have to match with the credentials saved in the database.
However, storing passwords as plain text in the database might not be a good approach. A database is open to many people in an organisation and it can be vulnerable to the outside world as well. So to secure passwords, hashing can be done before storing it in the database.
Hashing is a way to generate a fixed-size string from plain text. The output cannot be decrypted hence it’s “one-way” in nature making it highly secure. When we want to authenticate a user, we can create a hash of their password and then compare it to the stored hash in the database.
In this article, we will learn how to do password hashing in Node.js.
Also Read: Node Crypto Module – Encrypt and Decrypt Data
Node.js Bcrypt Module
In Node.js, we can use the bcrypt module to perform the hashing of sensitive information such as passwords. It provides an easy way to create and compare hashes.
Since bcrypt is not the Node.js default module we need to manually install it using NPM.
Installation and Importing Syntax
Below is the command to install bcrypt module in your project directory:
npm i bcrypt
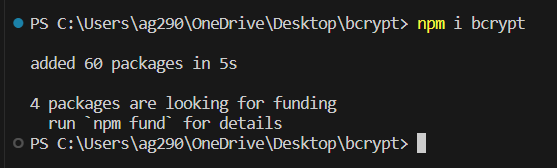
Below is the command to import bcrypt module in your JavaScript file in order to use its methods:
const bcrypt = require('bcrypt');

Once you have installed and imported the bcrypt module, you can use its method bcrypt.hash() for password hashing, let’s see how.
Hashing Password Using Node.js Bcrypt Module
Bcrypt provides both synchronous and asynchronous methods. The recommended one is the asynchronous method because hashing is CPU intensive, and the synchronous version will block the event loop and prevent your app from handling other requests until it finishes.
Below is the syntax for hashing using the asynchronous method. The first argument is the password and the second argument is the number of rounds (iterations) for the salt generation.
Syntax:
bcrypt.hash(plainText, saltRounds, function(err, hash) {
if (err) {
// Handle Error
} else {
// Handle Result
}
});
Example:
Below is an example utilising the above syntax to hash a password.
const bcrypt = require('bcrypt');
const plainText = 'this is a password';
const saltRounds = 10;
bcrypt.hash(plainText, saltRounds, function(err, hash) {
if (err) {
console.log(err);
} else {
console.log("Hash: " + hash);
}
});
Output:

Password Verification
As we mentioned earlier, for authentication, it is required to create a hash of the password and then compare it to the stored hash but with bcrypt module we can directly use its method bcrypt.compare() to compare plain text password with generated hash.
Below is the syntax for verifying the hashed password. The first argument is the plain password and the second argument is the hashed password to be compared.
Syntax:
bcrypt.compare(plainText, hash, function(err, result) {
if (err) {
// Handle Error
} else {
// Handle Result
}
});
Example:
Below is an example utilising the above syntax for password verification.
const bcrypt = require('bcrypt');
const plainText = 'this is a password';
const hash = '$2b$10$tw7PrZ2kK3c7u8HDB8du4epWIoIgJ.IAbA/77NjxjJs3pCBozwa1O'
bcrypt.compare(plainText, hash, function(err, result) {
if (err) {
console.log(err);
} else {
console.log("Match: " + result);
}
});
Output:

Conclusion
You must always salt and hash your password or any sensitive information that you don’t need in the original form again. Hashing is one way that means you can’t get the original data back from the hash but regenerate the same hash using the original data.
Password hashing in NodeJS can be easily done using bcrypt library. So, the next time you want to secure your users’ credentials, don’t forget to take advantage of its methods bcrypt.hash() and bcrypt.compare(). Also note that, in current days where there are so many advanced attacks happening such as brute-force attacks, hashes are not considered to be the most secure method, there are some other layers of security implementation & hashing algorithms that you should learn if you are writing production code.
Read More: Beginner’s Guide to Using Git and GitHub
Reference
https://www.npmjs.com/package/bcrypt