Welcome, coders! I hope you’ve gone through a complete guide on NumPy’s nanmax, and if you liked it, you’ll also enjoy this one and find it informative. In this article, we will learn how to detect the minimum values in a dataset containing NaN values. We will understand how this function works with some good examples, so let’s start.
Introducing numpy.nanmin
Numpy’s nanmin() function helps you find the smallest number in an array, even if there are “NaN” (Not a Number) values present. In real-world data, sometimes there are values missing, nanmin() doesn’t count those missing values when it’s figuring out the smallest number, so it gets the right answer.
It’s easy to use: you just put your numbers in, and it gives you the smallest one, even if there are missing values. Since it’s part of Numpy, it works well with other Numpy tools for analyzing data. And it doesn’t matter if your data is simple or complicated, nanmin() can handle it without any trouble.
Syntax of numpy.nanmin
Let’s take a closer look at the syntax of numpy.nanmin:
numpy.nanmin(array, axis=None, keepdims=False)
Here’s what each parameter means:
- array: This is the most important part of the function. It’s the list or array you want to find the smallest value in. You can use any kind of list or array-like thing, like a regular list or a Numpy array.
- axis=None: This part tells the function in which direction to look for the smallest value. If you don’t choose any direction (by setting it to None), the function checks the whole list or array. But if you want to check a specific direction, like rows or columns in a table, you can mention it here.
- keepdims=False: This decides if the size of the array should stay the same or not. By default, it’s set to False. If you leave it that way, the size related to the direction of the smallest value will shrink in the result. But if you set it to True, those dimensions will stay as they are.
How numpy.nanmin Works
As mentioned before, nanmin() function disregards NaN values and gives back the smallest number. It acts as if NaN values aren’t there when figuring out the smallest number.
For example, you have a list of temperatures recorded over a week, including some days where the temperature wasn’t recorded (NaN). You want to find the lowest temperature recorded during the week, but you want to ignore the days when the temperature wasn’t recorded. In this case, we use nanmin(). If the recorded temperature is [20, 25, 18, NaN, 22, 24, 19], nanmin() will return 18 as the answer.
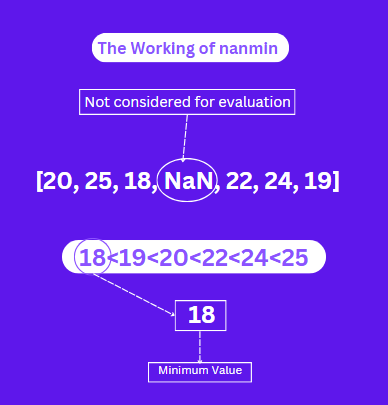
Picture that you have to work with a 2D array. In this case, you can use the axis parameter to specify how you want to calculate the minimum. If we specify axis=0, nanmin will find the minimum value along the columns (down the rows). For example, if we have data [[2, 3, NaN],[8, NaN, 5], [NaN, 1, 7]], then maximum values along the columns will be [2., 1., 5.]
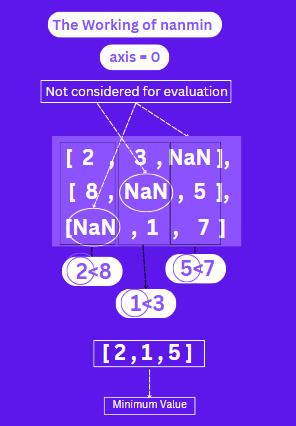
If we specify axis=1, nanmin will find the minimum value along the rows (across the columns). In this case, the minimum values along the rows are [2., 5., 1.]
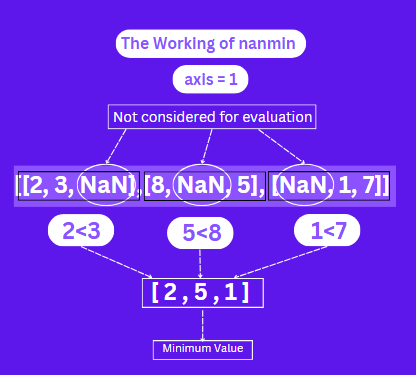
Using numpy.nanmin in Python
Since we’re clear on how the function works, let’s see how it performs in code.
Example 1: At first, we will use it with a 1-D array and find the minimum element among the given array.
import numpy as np
arr = [20, 25, 18, np.nan, 22, 24, 19]
result = np.nanmin(arr)
print("The smallest number in the given elements ", result)
Output:

Example 2: Now we will implement it with a 2-D array, specifying the axis to see how it works along both rows and columns.
import numpy as np
arr = np.array([[2, 3, np.nan],
[8, np.nan, 5],
[np.nan, 1, 7]])
min_col = np.nanmin(arr, axis=0)
print("Minimum value along columns:", min_col)
min_row = np.nanmin(arr, axis=1)
print("Maximum value along rows:", min_row)
Output:
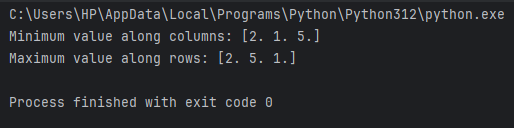
Example 3: If all values in the array are NaN, nanmin returns NaN.
import numpy as np
arr = np.array([[np.nan, np.nan, np.nan],
[np.nan, np.nan, np.nan],
[np.nan, np.nan, np.nan]])
min_col = np.nanmin(arr, axis=0)
print("Minimum value along columns:", min_col)
min_row = np.nanmin(arr, axis=1)
print("Maximum value along rows:", min_row)
Output:
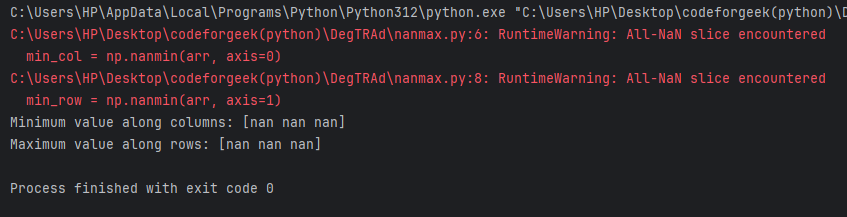
Example 4: Let’s see how it works with Positive and Negative Numbers
import numpy as np
data = np.array([2, -4, np.nan, 8, -12])
min_value = np.nanmin(data)
print("Minimum value (ignoring NaN values):", min_value)
nanmin looks at all numerical values, even negatives, and gives back the smallest one, ignoring any NaNs.
Output:
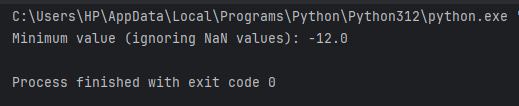
Summary
And that’s the end of this article. I hope this blog has cleared up your concepts about the nanmin function. Now you know which tool to use if you encounter NaN values, right? We covered the core workings of this function along with its demonstrations and use cases. If you enjoyed reading this one, don’t forget to check out how we can calculate the average of data in Python using NumPy.
Reference
https://numpy.org/doc/stable/reference/generated/numpy.nanmin.html