VM stands for Virtual Machine. A Virtual Machine is a virtual environment created in your pc which can run programs. It can be helpful to execute a peace of code in a different environment so that it doesn’t interrupt the main environment.
What is VM Module?
VM is a built-in module in NodeJS used to run JavaScript code on V8 Virtual Machine Contexts.
Syntax of VM Module in NodeJs
Below is the syntax to import the VM module in a NodeJS application.
const vm = require('vm');
Note: Do not use this module as a security mechanism to run anonymous code, the Virtual Machine Context can affect the actual server that why’s NodeJS Official Doc is also recommended not to run the untrusted code in that.
Example of VM Module in NodeJs
Below is an example that executes the code to increment the value of the count in a virtual machine context using the VM module.
const vm = require('vm'); // VM Instance
const contextObject = { count: 0 }; // Context Object
vm.createContext(contextObject); // Create Context
const code = 'count += 1;'; // Code to be Executed in the Context
vm.runInContext(code, contextObject); // Run the code in the Context of contextObject
console.log(contextObject); // Print the updated value
Output:

Methods of VM Module in NodeJS
VM Module has many methods, let’s see them one by one.
1. createContext()
This method can take an object as an argument to associate with a new instance of a V8 context and return the contextified object, we can perform operations on contextified object using the runInContext() method.
Syntax:
createContext([contextObject[,options]]);
where
- contextObject is the object to be contextify,
- options is an optional value
Example:
const vm = require('vm');
const contextObject = { count: 0 };
const contestifiedObject = vm.createContext(contextObject);
console.log(contestifiedObject);
Output:
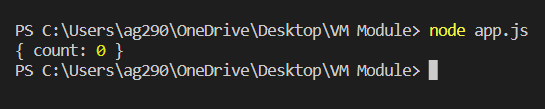
2. runInContext()
This method is used to run a code statement on the contextifiedObject created by the createContext() method. This method takes a code in a string to execute, a contextified object, and options as an argument.
Syntax:
vm.runInContext(code, contextifiedObject[,options]);
where
- code is a code statement inside a string
- contextifiedObject is a contestified object,
- options is an optional argument such as filename, lineoffset, columnoffset, etc.
Example:
const vm = require('vm');
const contextObject = { count: 0 };
const contestifiedObject = vm.createContext(contextObject);
const code = 'count += 1;';
vm.runInContext(code, contestifiedObject);
console.log(contestifiedObject);
Output:

3. runInNewContext()
This method can create a contextified object and run the code in that context simultaneously. It is not required to use createContext() method to create a contextified object to run the code unlike runInContext() method where is it required to have a contestifed object to run the code in it.
Syntax:
vm.runInNewContext(code[,contextObject[,options]]);
where
- code is a code statement inside a string,
- contextObject is the object to be contextify,
- options is an optional argument such as filename, lineoffset, columnoffset, etc
Example:
const vm = require('vm');
const contextObject = { count: 0 };
// const contestifiedObject = vm.createContext(contextObject); not required
const code = 'count += 2;';
vm.runInNewContext(code, contextObject);
console.log(contextObject);
Output:

4. runInThisContext()
This method run the code in the current context and returns the output.
Syntax:
vm.runInThisContext(code[,options]);
where
- code is a code statement inside a string,
- options is an optional argument such as filename, lineoffset, columnoffset, etc
Example:
const vm = require('vm');
const localVar = 1;
const result = vm.runInThisContext('localVar = 2');
console.log("Local Variable: " + localVar);
console.log("Output: " + result);
Output:
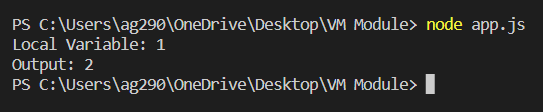
Here you have noticed that the local variable is unchanged because the runInThisContext() method has no access to the local scope.
5. isContext()
This method is used to verify whether an object has been contextified or not. It returns true if the object passed as an argument to this method is contextified.
Syntax:
isContext(object)
where
- object is the object to check whether it is contextified or not.
Example:
const vm = require('vm');
const contextObject = { count: 0 };
vm.createContext(contextObject);
const result = vm.isContext(contextObject);
console.log(result);
Output:
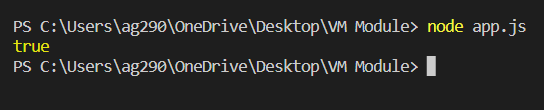
Summary
VM Module is a special type of NodeJS built-in module to compile and runs code in a Virtual Machine Context. VM module has various methods to create context objects, run code on the context, check for the contextified object, etc. Hope this tutorial helps you to understand the VM Modules and their methods in NodeJS.
Reference
https://nodejs.org/api/vm.html