NodeJS Net is a built-in module used to create servers and clients. It can be used in different types of applications to create a connection between servers and clients, it acts as a bridge of communication between servers and clients. Beside this Node.js Net provides different methods used to validate the IP address, to check the IP address type i.e IPv4 or IPv6.
For a better understanding, let’s consider an example, suppose we have to create a group chat application, and we have to create different groups for different users, here the group is considered a server and users are clients connected to different groups, so we can use Node.js Net module to create different servers on a different port and give users the option to choose which server they want to connect, and as per the choice, create a client using the Net module of that server which use to connect with the server.
Also read: NodeJS Timer Module and Methods to Know
Node.js Net Module
For using Node.js Net functionalities, it is required to import Net Module using the following code.
Syntax:
const net = require('net');
Create Server using Node.js Net Module
Node.js Net provides a method used to create a new server.
Syntax:
net.createServer();
Example:
Let’s create a server and set it to a constant then use that constant and create a port using the listen method to listen to the server, here we specified the port as 3000, and print a string to confirm whether the server is successfully created or not.
const net = require('net');
const server = net.createServer();
server.listen(3000, () => {
console.log('Server listening on port 3000!');
});
Output:
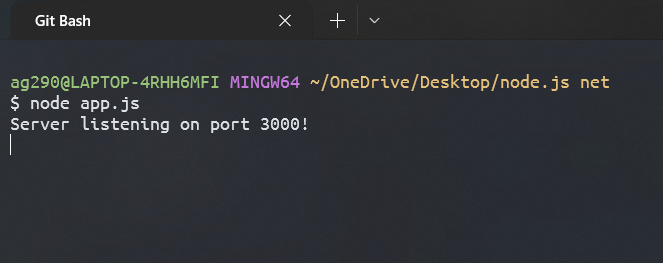
Create Client using Node.js Net Module
There are two methods provided by Net Module to create a client, the first is connect and the second is createConnection, but both methods are completely identical, they have the same definition.
connect
Syntax:
net.connect(port, callback);
Example:
Let’s use the connect method to connect with the server we have created in the above step.
const net = require('net');
const server = net.createServer();
server.listen(3000, () => {
console.log('Server listening on port 3000!');
});
net.connect(3000, () => {
console.log('connected to server!');
});
Output:
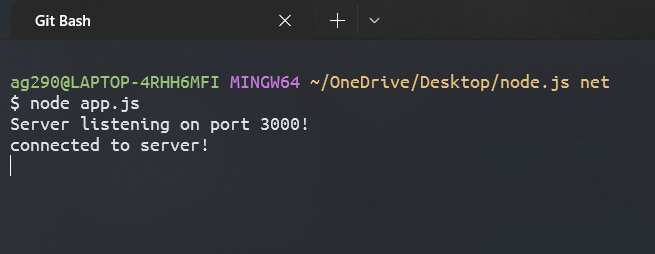
createConnection
Syntax:
net.createConnectiont(port, callback);
Example:
To see the difference between connect method and createConnection method, use the same example by just changing the keyword connect to createConnection.
const net = require('net');
const server = net.createServer();
server.listen(3000, () => {
console.log('Server listening on port 3000!');
});
net.createConnection(3000, () => {
console.log('connected to server!');
});
Output:
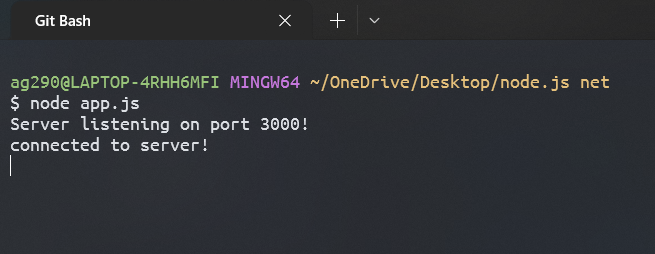
Methods of the Node.js Net module
There are methods used for IP address validation, it detects whether the entered IP address is correct or not, whether it is an IPv4 or IPv5.
isIP
This method takes a string as an argument and checks whether it is a valid IP address or not, it is not a valid IP address then the method returns 0 as output.
Syntax:
net.isIP("IP Address")
Example:
const net = require('net');
const result = net.isIP("8.8.8.8");
if(result) {
console.log("It's an IP address");
} else {
console.log("It's not an IP address");
}
Output:
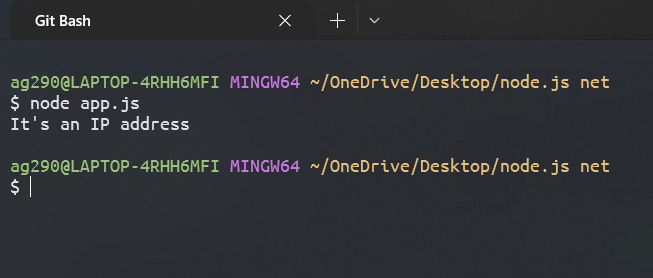
isIPv4
This method takes a string as an argument and checks whether the entered IP address is an IPv4 or not. IPv4 is developed in the 90s and has 4 billons addresses used by the majority of websites. This method returns a true if the argument passed is an IPv4, and returns a false if it is not IPv4.
Syntax:
net.isIPv4("IP Address")
Example:
const net = require('net');
const result = net.isIPv4("8.8.8.8");
if (result) {
console.log("It's an IPv4 address");
} else {
console.log("It's not an IPv4 address");
}
Output:
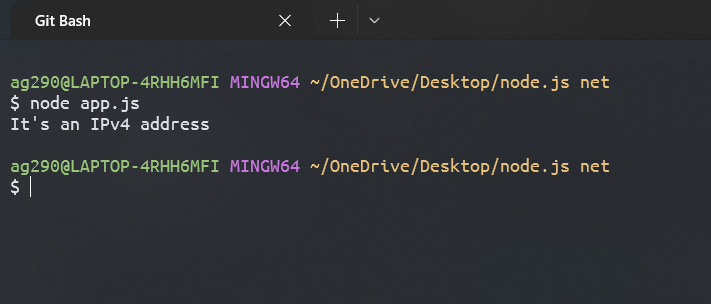
isIPv6
This method works the same as the isIPv4 method but instead of checking for the IPv4, it validates whether the entered IP address is IPv6 or not. The number of IPv4 addresses is limited that why IPv6 is created which is used by new websites.
Syntax:
net.isIPv6("IP Address")
Example:
const net = require('net');
const result = net.isIPv6("8.8.8.8");
if(result) {
console.log("It's an IPv6 address");
} else {
console.log("It's not an IPv6 address");
}
Output:
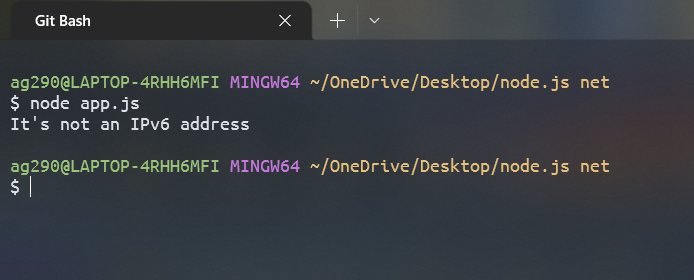
Summary
Node.js Net module is used to create servers and clients for that server, it is mainly used in chat applications where we usually have to create many servers and clients which connect to share information, Node.js Net will create that connection, it can also use for checking the IP address types and to validate a string that it is an IP address or not. Hope this article helps you to understand the Node.js Net module.
Reference
https://nodejs.org/api/net.html#net