MongoDB database has one or many collections in which records are present. These records are called documents in MongoDB.
Overview of MongoDB Sorting
Sorting is a technique to rearrange documents. This rearrangement can be ascending or descending according to the requirements.
A real-life example is the student records, they are arranged in ascending order by name. Suppose a school has hundreds of records of students stored in the MongoDB database and new students are added continuously, it is hard to maintain the order, so MongoDB sorting is used to sort the records of students in ascending order of their names.
Sorting can be directly performed when selecting the documents from a collection. We have a separate article on selecting documents from a collection if you want to read it.
Sorting Documents with the sort() Method
This method is used for shorting the documents in ascending or descending order.
This method takes an object as an argument containing the key-value pair. The key is the field on which the documents will sort and the value defines whether to sort in ascending or descending order.
Syntax:
sort({key: value})
where
- key is a field by which the documents will sort,
- value decide whether to sort in ascending or descending
Sort in Ascending Order
For shorting documents in ascending order, we have to pass the value as 1.
Syntax:
database.collection(collectionName).find({}).sort({key: 1}).toArray(function(err, sortDocuments) {
if (err) throw err;
console.log(sortDocuments);
});
where
- database is a database object,
- collectionName is the name of a collection,
- key is a field by which the documents will sort
Example:
Let’s see an example to sort the documents in ascending order. We have multiple documents inside a collection.
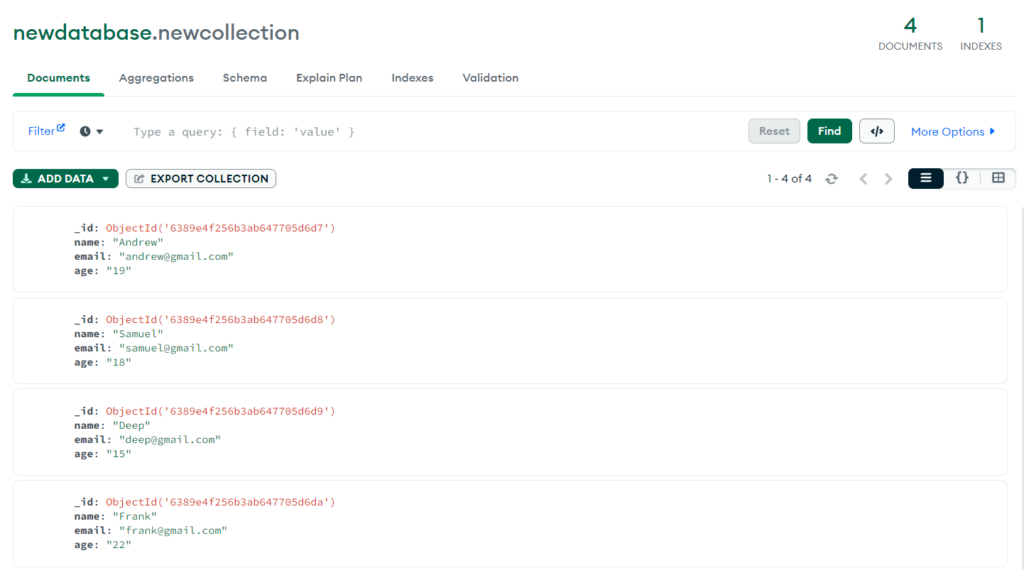
Below is the code to sort them in ascending order of age field.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
database.collection("newcollection").find({}).sort({age: 1}).toArray(function(err, documentsArray) {
if (err) throw err;
console.log(documentsArray);
});
});
Run the Code:
Create a folder, and copy and paste the above code into a JavaScript file “app.js”. Then locate the folder in the terminal and run the below command to install the MongoDB module.
npm install mongodb
Run the below command to run the code.
node app.js
Output:
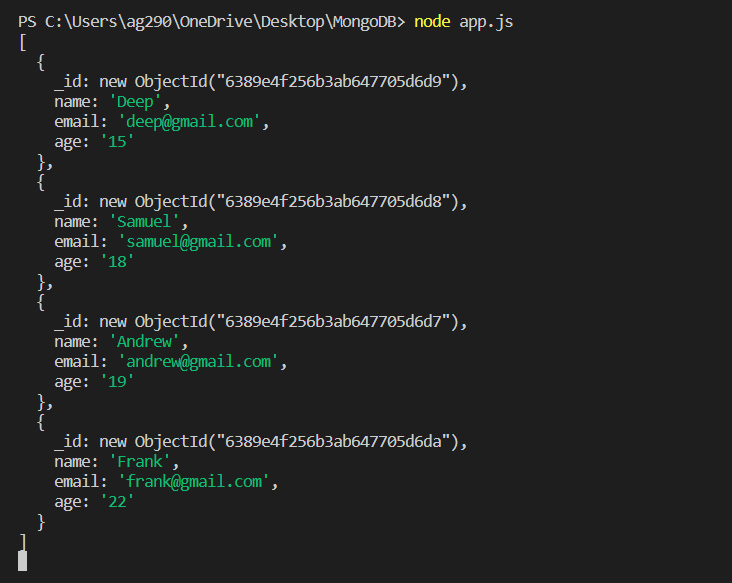
In the above output, you can see that the documents are sorted ascendingly according to age.
Sort in Descending Order
For shorting documents in descending order, we have to pass the value as -1.
Syntax:
database.collection(collectionName).find({}).sort({key: -1}).toArray(function(err, sortDocuments) {
if (err) throw err;
console.log(sortDocuments);
});
where
- database is a database object,
- collectionName is the name of a collection,
- key is a field by which the documents will sort
Example:
Below is the code to sort the documents in descending order of age field.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
database.collection("newcollection").find({}).sort({age: -1}).toArray(function(err, documentsArray) {
if (err) throw err;
console.log(documentsArray);
});
});
Run the Code:
Create a folder, and copy and paste the above code into a JavaScript file “app.js”. Then locate the folder in the terminal and run the below command to install the MongoDB module.
npm install mongodb
Run the below command to run the code.
node app.js
Output:
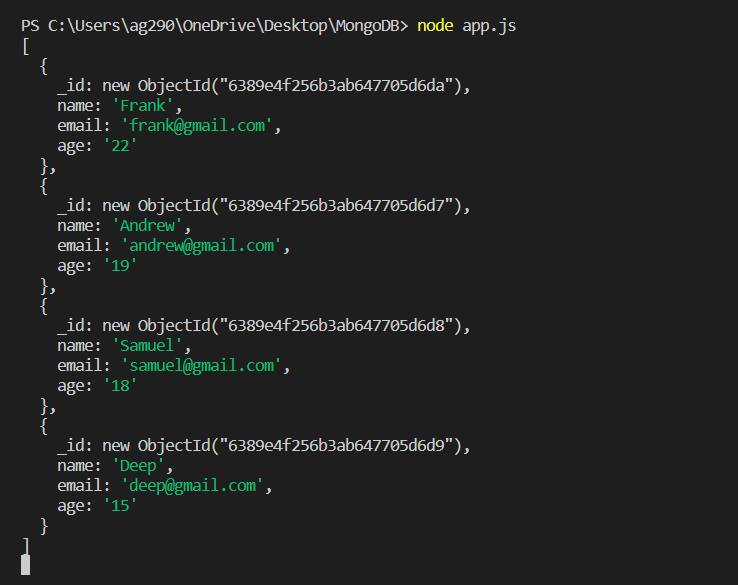
In the above output, you can see that the documents are sorted descendingly according to age.
Sorting Documents by Characters
In the above example, we have sorted the documents on the basis of the age field which is obviously a number. But we can also perform sorting on the basis of characters.
Example:
Below is an example to sort the documents on the basis of the name field in ascending order.
const { MongoClient } = require('mongodb');
MongoClient.connect("mongodb://localhost:27017/", function (err, client) {
if (err) throw err;
const database = client.db("newdatabase");
database.collection("newcollection").find({}).sort({name: 1}).toArray(function(err, documentsArray) {
if (err) throw err;
console.log(documentsArray);
});
});
Output:
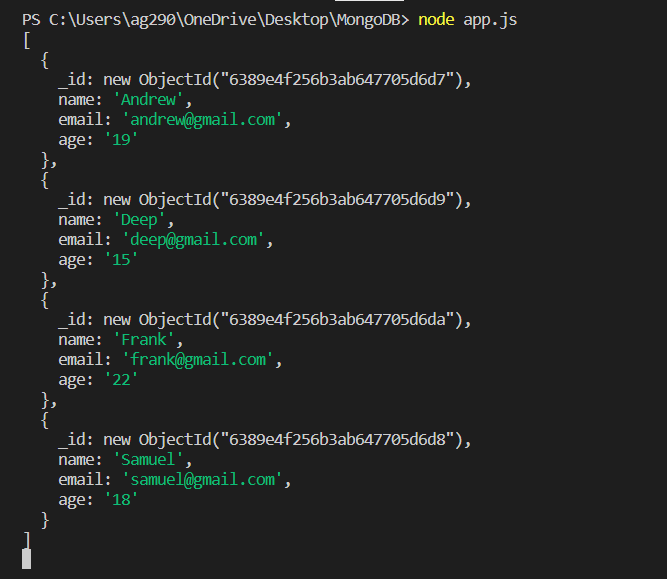
In the above output, the documents are sorted in ascending order by name.
Summary
Sorting is a process of rearranging documents when selecting from a collection. This rearrangement can be ascending or descending according to the requirement. For sorting documents in NodeJS, there is a method sort() which can use with find() methods and take an object containing a key and a value as an argument to sort the documents. If the value is ‘1’ then it will sort documents in ascending order and if it is ‘-1’ then in descending order. Hope this tutorial helps you understand the sorting of MongoDB documents in NodeJS.
Reference
https://www.mongodb.com/docs/drivers/node/current/fundamentals/crud/read-operations/sort/