Variables are essential for storing values, creating functionalities, and building the application. When we use variables in an application, it is present in the code, right? The code is always associated with the application, and any user can see that code. For example, you are creating a front-end application, having a variable in JavaScript to store some crucial information, but anyone who has access to the application can see that variable. Now, this lets to a privacy attack and can be a major security issue. The solution is using the Environment Variables.
Before diving deep into this concept, it is required to have prior knowledge of variables, we recommend you read our articles on JavaScript Variables because it is precisely the same as in Node.js.
Environment Variables
The variables that are present or created outside of a context of a program for providing security on credentials to streamline the application are called environment variables.
Example:
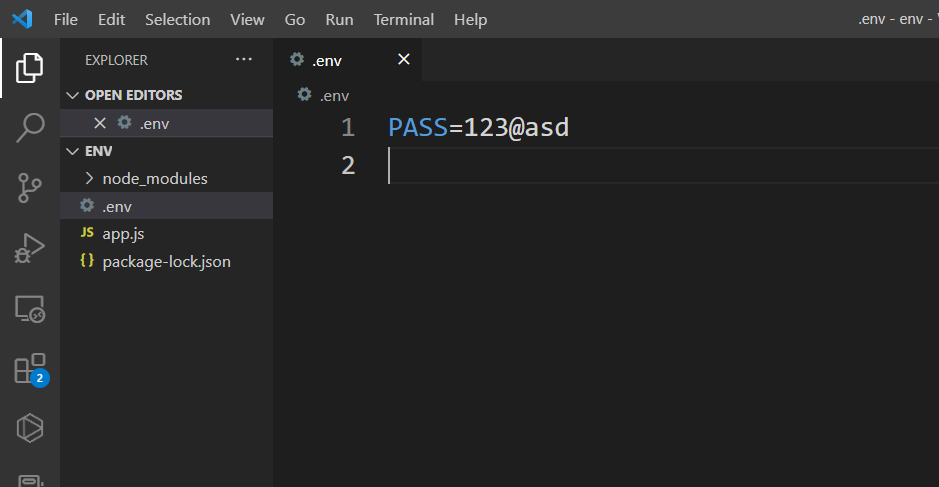
Here we have declared an environment variable PASS that contains 123@asd as a value. This environment variable is not accessible in the code, it gets loaded into the process object when the application runs.
Setting Environment Variables in Node.js
For setting the environment variables, creating a file “.env” is required, where these variables are initiated. The name and value of the environment variables are separate with equal(=) and it is recommended to avoid using unnecessary whitespace anywhere.
Syntax:
ENVIRONMENT_VARIABLE_NAME=VALUE
where
- ENVIRONMENT_VARIABLE_NAME is the environment variable’s name
- VALUE is the value that this variable contains
Note: Do not upload this file anywhere like on GitHub, or any other hosting platform otherwise there would be no point in creating environment variables.
Example of Setting Environment Variables in Node.js
Here we have a simple Node.js application having a single file “app.js”, this file contains console.log(Name) which prints the value of NAME variables in the console.
app.js
console.log(NAME);
Let’s create a “.env” file, and initialise NAME variables to a value “Hello World!”.
NAME=Hello World!
Now to run the program, execute the below command in the terminal.
node app.js
Output:
console.log(NAME);
^
ReferenceError: NAME is not defined
at Object.<anonymous> (C:\Users\ag290\OneDrive\Desktop\env\app.js:3:13)
at Module._compile (internal/modules/cjs/loader.js:759:30)
at Object.Module._extensions..js (internal/modules/cjs/loader.js:770:10)
at Module.load (internal/modules/cjs/loader.js:628:32)
at Function.Module._load (internal/modules/cjs/loader.js:555:12)
at Function.Module.runMain (internal/modules/cjs/loader.js:826:10)
at internal/main/run_main_module.js:17:11
We have an error “NAME is not defined” since the environment variables are not directly accessible in the code, it only gets loaded in the process.env when the application runs.
Accessing Environment Variables in Node.js
To access the environment variables we have to use a process object that has an env that contains all the environment variables.
Syntax:
process.env.ENVIRONMENT_VARIABLE_NAME
where
- ENVIRONMENT_VARIABLE_NAME is the name of the environment variable you want to access
Use the above statement to access environment variables in the program.
Example of Accessing Environment Variables in Node.js
Extending the above example, let’s use process.env.NAME to access the value of NAME variables.
Before that, it is also required to install a module “dotenv”. Execute the below command in the terminal to install this module in the project folder.
npm i dotenv
Here we have used NPM to install “dotenv” module.
Then use require to important this module and config, now we can use process.env.NAME to get the value of NAME variables
app.js
const env = require('dotenv').config();
console.log(process.env.NAME);
Again run the program using the below command.
node app.js
Output:
Hello World!
See, we now have access to Environment variables.
Summary
Environment Variable in Node.js is useful to store credentials, these are initiated outside of the program in a separate “.env” file. These environment variables can be assessed using the Node.js process object. Hope this tutorial helps you to understand the environment variable in Node.js.
Reference
https://stackoverflow.com/questions/22312671/setting-environment-variables-for-node-to-retrieve
https://nodejs.org/dist/latest-v8.x/docs/api/process.html