Node.js DNS is used to get information regarding the DNS by doing a DNS lookup.
DNS Lookup means looking at the DNS records for a domain or an IP address of a server. These DNS records can be the list of different IP addresses, MX records, Name Server records, or services associated with a particular domain. Not only this there are various functionalities provided by the DNS module, which we will understand in this article.
What is DNS?
Before going further let’s try to understand the concept of DNS for better understanding. Each system connect to the internet has its address which is called an IP address. DNS stands for Domain Name System used to convert the hostname into an IP address.
Also read: NodeJS Command Line Options
The NodeJS DNS Module
Node.js DNS can be used by importing the DNS modules using the following statement.
Syntax:
const dns = require('dns');
DNS Module Methods
The DNS module provides various methods let’s understand them one by one.
lookup()
This method of DNS module is used to find the IP address of a domain name.
Syntax:
dns.lookup('domain', (err, result) => {
// function body
})
This method takes two arguments, a domain to find its IP address, and a callback function, in which we can print the error or the IP of the entered domain.
The lookup method is used a computer internal system to get the IP address of the entered domain.
Example:
const dns = require('dns');
dns.lookup('askpython.com', (err, result) => {
if(err) {
console.log(err);
} else {
console.log(result);
}
})
Let’s use this function to find the IP address of “askpython.com”, and inside the callback function first check for the error if the error occurs print it in the console, if not then print the returned IP address.
Output:
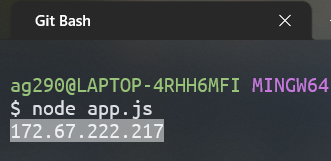
loopupService()
This method takes three augments, first is the IP address, second is the Port and third is a callback function that returns the host main and service associated with it.
Syntax:
dns.lookupService('domain', port, (err, host, service) => {
// function body
})
resolve()
The resolve method is also used to get the IP address of an entered domain, but instead of using the computer’s internal system just like the lookup method to find the IP, it makes a network request to the entered domain name and then finds all the IP address link with that domain.
Syntax:
dns.resolve('domain', (err, result) => {
// function body
})
Example:
Let’s use the same example to see the difference between the output of the lookup method and the resolve method.
const dns = require('dns');
dns.resolve('askpython.com', (err, result) => {
if(err) {
console.log(err);
} else {
console.log(result);
}
})
Output:
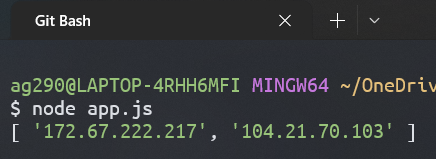
There are various types of resolve method which work similarly, but returns the array with a certain type of IP address. Some of them are –
- resolve4()
This method returns an array of IPv4 IP addresses which is the older version of the IP address. IPv4 is developed in the 90s and has 4 billons addresses used by the majority of websites.
- resolve6()
This method returns an array of IPv6 IP addresses which is the newer version of the IP address. Since the number of IPv4 addresses is limited, IPv6 is created which is used by new websites.
- resolveMx()
This method returns an array of MX records associated with the entered domain. MX is a Mail Exchange record.
- resolveNs()
This method returns an array of NS records. NS stands for Name Server, you may hear this word when linking a domain to a hosting server.
reverse()
This is just the opposite of the lookup() and resolve() method, this method takes the IP address as an argument and returns the domain associated with that IP address.
Syntax:
dns.reverse('ip-address', (err, result) => {
// function body
})
Example:
Let’s use this method and put the google public IP address, to see the result.
const dns = require('dns');
dns.reverse('8.8.8.8', (err, result) => {
if(err) {
console.log(err);
} else {
console.log(result);
}
})
Output:
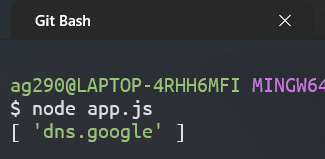
Summary
Node.js DNS provides many useful methods to get information regarding the domain and the IP address. By using it you can find the IP address associated with a domain, and you can also find the services associated with a particular IP. It also helps in finding the Name Server and Mail Exchanges record of a domain. Hope this article helps you to understand the DNS module of Node.js.
Reference
https://nodejs.org/api/dns.html#dns