JavaScript allows to perform mathematical operations on strings without throwing an error or warning, but the result may not be the expected result, so it is suggested to first convert it into an integer. The strings in JavaScript can be converted into integers using various methods.
This tutorial will cover different methods for converting a string containing a number into an integer.
Problem Statement
Below is the code containing a number inside a string, then two operations perform on it, the first is to divide by 2, and the second is to add 2 and print the result in the console.
const str = '10';
const divide = str / 2;
const sum = str + 2;
console.log("Divide: " + divide);
console.log("Sum: " + sum);
Output:

Here the JavaScript performs division operations properly, but instead of adding value, it concatenates them, because it is considered as a string.
The flexibility of assigning an integer to a string can spoil the mathematical operations and break the consistency of the code, so it is necessary to convert the unknown value into an integer and then perform any operation.
Method to Convert String To Int in NodeJS
JavaScript has many methods to convert a string into an Integer, let’s see them one by one.
parseInt()
This method takes a string and radix as an argument, radix is an option argument representing the base in a numerical system such as 2 for binary, 10 for decimal, etc.
Example:
const str = '10';
const num = parseInt(str);
const divide = num / 2;
const sum = num + 2;
console.log("Divide: " + divide);
console.log("Sum: " + sum);
Output:
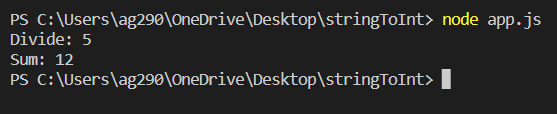
Validate:
To validate that the data type of output is a number, we can use the typeof() method.
const str = '10';
const num = parseInt(str);
console.log(typeof(num));
Output:

parseFloat()
This method is similar to parseInt(), the only difference is the patrseInt() method converts the string of float value into an integer whereas parseFloat() converts it into float.
Example:
const str = '10.25';
const num = parseFloat(str);
console.log(num);
Output:

Math.floor()
This method is also used to convert a string into a number rounded down to the nearest integer.
Example:
const str = '10.25';
const num = Math.round(str);
console.log(num);
Output:

Math.ceil()
This method converts a string into a number and returns the number round to the nearest integer.
Example:
const str = '10.25';
const num = Math.ceil(str);
console.log(num);
Output:

Number()
This method can take a string as an argument, convert it into a number, and return it.
Example:
const str = '10';
const num = Number(str);
console.log(num);
Output:

Mathematical Operation
Mathematical operations like multiplication, division, and subtraction convert a string into a number in JavaScript, so we can take the advantage of this JavaScript property to make it super easy to convert a string into a number, let’s see one by one how we can do this using the different operator.
Multiplying by 1
A string can be multiplied by the number 1 to convert it into a number.
Example:
var x = '10';
console.log(x + ' is ' + typeof(x));
x = x * 1;
console.log(x + ' is ' + typeof(x));
Output:

Dividing by 1
Diving a string by the number 1 can be used to convert it into a number.
Example:
var x = '8';
console.log(x + ' is ' + typeof(x));
x = x / 1;
console.log(x + ' is ' + typeof(x));
Output:

Subtracting 0
A string can be converted into a number by subtracting the number 0 from that string.
Example:
var x = '6';
console.log(x + ' is ' + typeof(x));
x = x - 0;
console.log(x + ' is ' + typeof(x));
Output:

Unary Plus Operator
The simplest way to convert a string into a number is to use a plus operator at the beginning of the string.
Example:
var x = '10';
x = +x;
console.log(typeof(x));
Output:

Summary
Converting a String to an Integer is essential to perform a bug-free mathematical calculation. If we try to perform a mathematical operation on a string, JavaScript does it without throwing an error, but the output may not be the required output, so it is recommended to convert an unknown value to an integer before performing any operation on it. Hope this tutorial helps you to convert a string into an integer in JavaScript.
Reference
- https://stackoverflow.com/questions/1133770/how-to-convert-a-string-to-an-integer-in-javascript
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/parseInt