Have you ever thought of creating a static website from your Next.js application? Yes, one of the coolest features of Next.js is the ability to export HTML/CSS/JS together and they work exactly the same as in the Next.js app and can be deployed to any static hosting service. Well, let’s see in detail how it is possible and how to do it.
Why Export as a Static Website?
There are various reasons to export Next.js as a static website:
- Improved Performance: Static websites render content quickly because the HTML files are pre-generated and served directly to users. This eliminates the need for server-side rendering on each request, hence improving the overall performance.
- Cost-Efficiency: Hosting static files is less costly as compared to buying a server to host the whole Next.js app. Even lots of platforms offer free hosting for static websites like Netlify, GitHub, etc.
- Simplicity: Deploying and managing static files is easy, so no need for high technical knowledge.
- Testing: Exporting static files from Next.js is also useful for testing purposes or to show a demonstration of the actual website to the client.
Exporting Pure HTML/CSS/JS from Next.js
Let’s see the step-by-step process to export a Next.js application.
Step 1: Creating a New Next.js Project
Let’s start with creating a new Next.js application:
npx create-next-app@latest
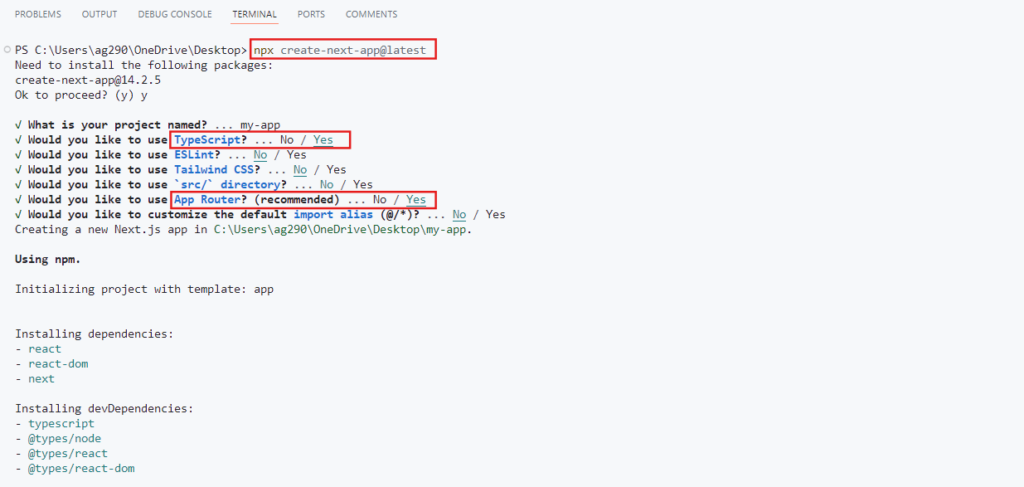
Check Yes for both TypeScript and App Router to follow along with us.
If you want a detailed guide on how to start with Next.js, click here.
Step 2: Creating Different Pages
Now open the project inside a code editor, delete all the files in the app directory except layout.tsx and create the following files:
app/page.tsx:
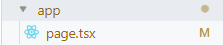
const HomePage = () => {
return <h1>Home Page</h1>;
};
export default HomePage;
This page is for the home page, which means when someone tries to access the ‘/’ route, this will open.
app/about/page.tsx:
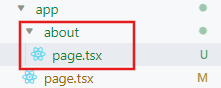
const AboutPage = () => {
return <h1>About Us</h1>;
};
export default AboutPage;
This will create another page which will open for the URL ‘/about’.
app/contact/page.tsx:
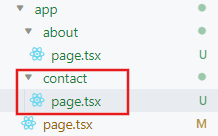
const ContactPage = () => {
return <h1>Contact Us</h1>;
};
export default ContactPage;
This page will open for the URL ‘/contact’.
So eventually we created three pages: home (“/”), about (“/about”) and contact (“/contact”). This process is called file-based routing which we have covered in another tutorial.
Step 3: Changing Output Mode to Export
It’s time for configuration. Open ‘next.config.js’ or ‘next.config.mjs’, whatever you have in your project directory and change the output mode to export.
output: 'export'
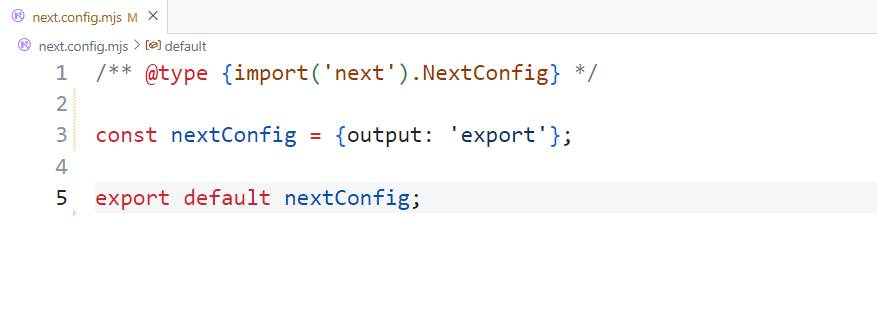
Step 4: Executing the Build Command
Now inside the terminal, execute the below command to run ‘next build’.
npm run build
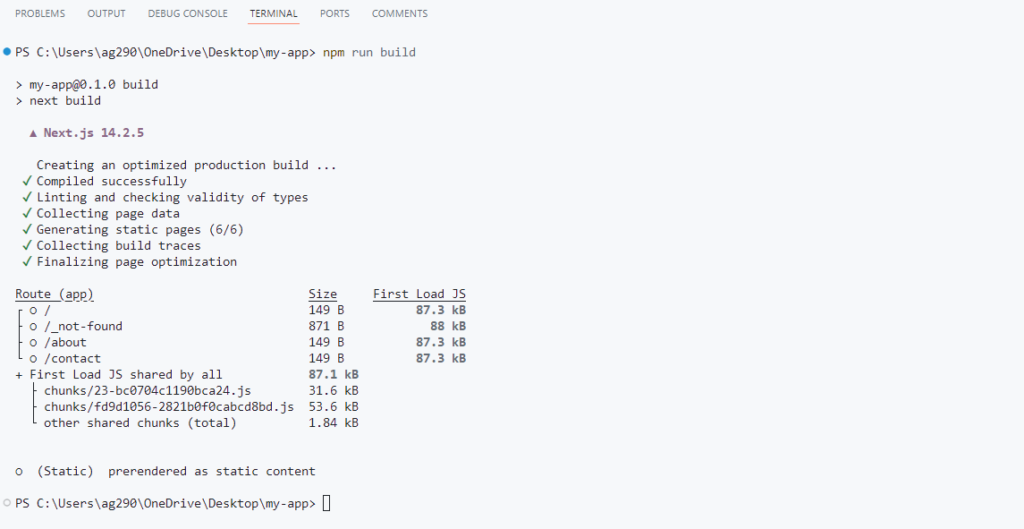
Also as we changed the output mode, this command will create an out folder having HTML/CSS/JS assets from the Next.js app we just created.
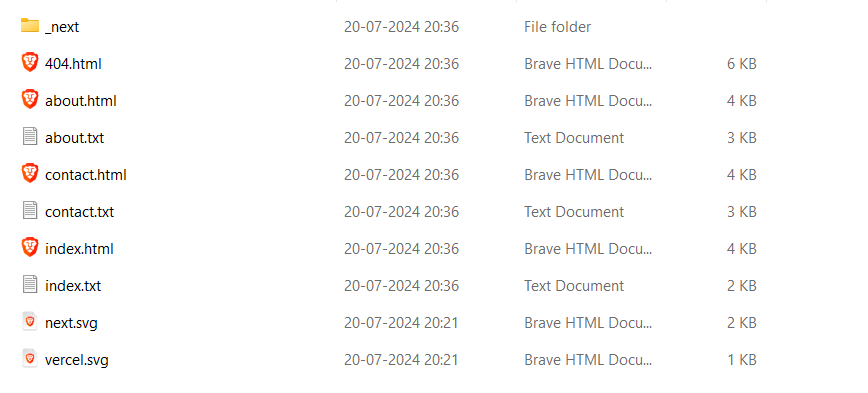
Now as we can see, all our pages along with the 404 page that Next.js generates by default get exported to out folder. You can now host them as static pages wherever you want.
Summary
Optimizing Next.js is really an important part of development and we already know many techniques to achieve this like using built-in components (<Link>, <Image>, <Script>, etc), removing unused packages, checking bundle sizes, etc. However, generating static HTML from it is somewhat new and useful. It saves the cost which is more when hosting a full stack project. It will also help in faster rendering and ultra level of optimization as a static HTML page is highly optimized compared to any kind of technique, and it is very lightweight.
To export static assets in Next.js, we just have to change the output mode to export and execute the next build command and we got a folder out with all the static assets.
Reference
https://nextjs.org/docs/app/building-your-application/deploying/static-exports