Node.js is an emerging technology, and with Express it become insanely powerful. We can use Node.js with Express to manage sessions. In this tutorial, you will learn to manage sessions including setting up sessions, saving data in sessions and fetching data from sessions.
A website uses HTTP protocol to transfer information between a client and a server. This HTTP protocol is a stateless protocol, where the server won’t have a status of communication and it doesn’t keep track of requests and responses made from a client to the server. In terms of security, it is really better to track the user request to know how many times a user requests or which data a user wants to access, etc.
To make the HTTP protocol stateful from stateless, we have sessions.
Session in Node.js
A session comes into action when a client makes a request to the server, a server then creates a session with a unique ID sent to the user as a response as cookies and stored on their browser and the session server created is stored in the server. Next time when the same user comes, the session unique ID attached to the user will also come and the server knows that this user is the same user as the previous one.
For creating a session in Node.js, we have a module “express-session”.
express-session Module in Node.js
This module is used to manage sessions in Node.js, for installing this module in your Node.js project using NPM, execute the below command in the terminal.
npm i express-session
If you got an error, make sure that you have Node and NPM installed on your system.
Session Setup in Node.js
To use this “express-session” module to set a session in Node.js, it is also required to install the Express module. Execute the below command in the terminal to install Express.
npm i express
Once you have installed the express-session and express module, it’s time to create a server file “app.js” to set up the session.
Inside the server file, let’s start with importing the express and express-session module, then create an instance of express and use it to call the “use” method and pass the session method with an object as an argument containing a secret key, and some optional parameters.
const express = require('express')
const session = require('express-session')
const app = express()
app.use(session({secret: 'Your_Secret_Key', resave: true, saveUninitialized: true}))
Once we have set up the session, it’s time to create session variables, to store some information.
Creating Session Variables
Below is the syntax to create session variables:
req.session.key = value
where the key is later used to access the value.
Let’s create a variables userName and store some values in it.
app.get("/", function(req, res){
req.session.userName = 'Aditya@123';
res.send("Thanks for visiting");
})
Accessing Session Variables
Below is the syntax to Access session variables:
req.session.key
Let’s access the session variables userName which we have created in the above step, and send its value as a response to the client.
app.get("/login", function(req, res){
var userName = req.session.userName;
res.send("Welcome " + userName);
})
Complete Code:
const express = require('express')
const session = require('express-session')
const app = express()
app.use(session({secret: 'Your_Secret_Key', resave: true, saveUninitialized: true}))
app.get("/", function(req, res){
req.session.userName = 'Aditya@123';
res.send("Thanks for visiting");
})
app.get("/login", function(req, res){
var userName = req.session.userName;
res.send("Welcome " + userName);
})
app.listen(3000, function(error){
if(error) {
console.log(error)
}
console.log("Server listening on PORT 3000")
})
Run the Application
The application code is written in a single file “app.js” that runs on executing the below command in the terminal.
node app.js
Open the Application
Enter the below URL inside the search bar of a web browser to open the application.
http://localhost:3000/
Output:
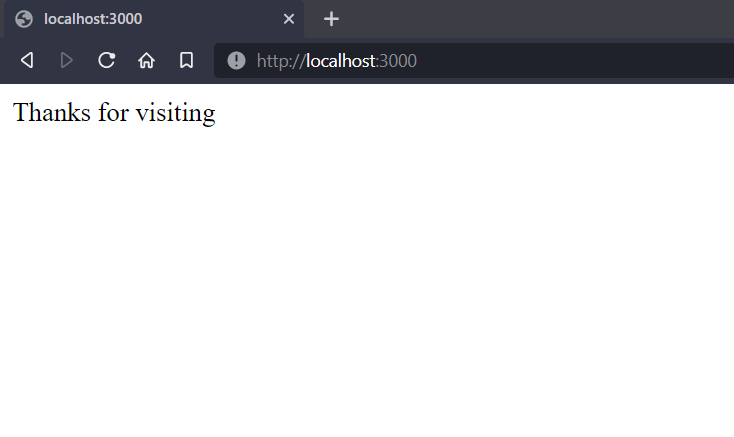
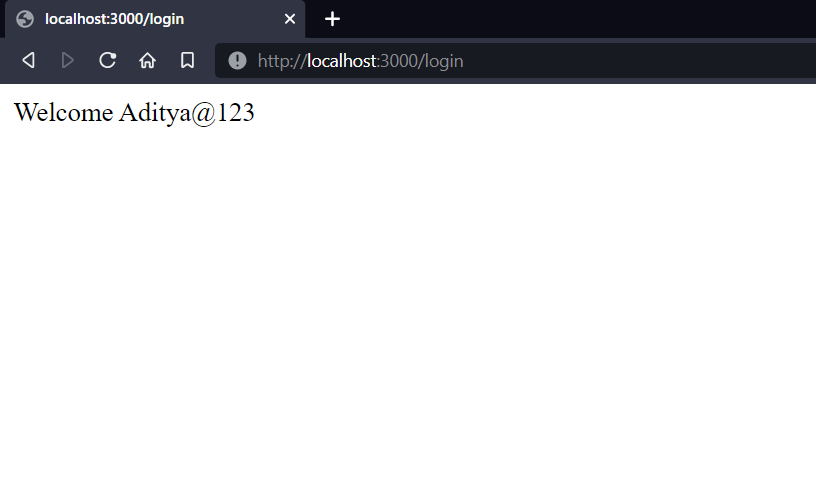
Summary
The session is important to keep track of its clients, to have knowledge about how many times they request the server and what response they want, etc. It provides security as well. For setting sessions, we have a module express-session which can be installed using NPM. Hope this tutorial helps to understand the way of managing sessions using Node.js and Express.
Reference
https://www.npmjs.com/package/express-session