Developers use javascript:void(0) in hyperlinks to prevent the browser default action, like navigating to a new page. The void operator makes sure that the link returns undefined, stopping the page from reloading or changing when clicked. In this article, let’s understand this in the simplest way possible.
What Is javascript:void(0)
Let’s break the word “javascript:void(0)” to understand it’s meaning clearly:
- javascript: This tells the browser that we are about to run some JavaScript code.
- void(0): This means “do nothing” or “return nothing”.
So, when we use javascript:void(0), it just asks the link to eat Cadbury 5-Star and do nothing, or we can say that it stops the browser from following the link or reloading the page.
Why Use javascript:void(0) in Links
Sometimes, developers need links that do more than just navigate to another page. For example, they might want the link to:
- Show a hidden section of the page, like a dropdown menu.
- Trigger an action, such as to open a popup or to submit a form.
- Run a small script, like to change the text on the page or to show a message.
In these cases, they don’t actually want the link to take the user to a different web page.
Now, if we run a link using the ‘#’ attribute and show an alert after the link is clicked, like this:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Submit Link Example</title>
<script>
function showAlert() {
alert("Your response is submitted");
}
</script>
</head>
<body>
<a href="#" onclick="showAlert()">Submit</a>
</body>
</html>
Then it might cause some problems like:
- The page could scroll to the top if # is used as the link’s href attribute. This happens because # tells the browser to go to the top of the current page.
- The link may refresh or reload the page, even though the developer just wants to run some code.
Output:
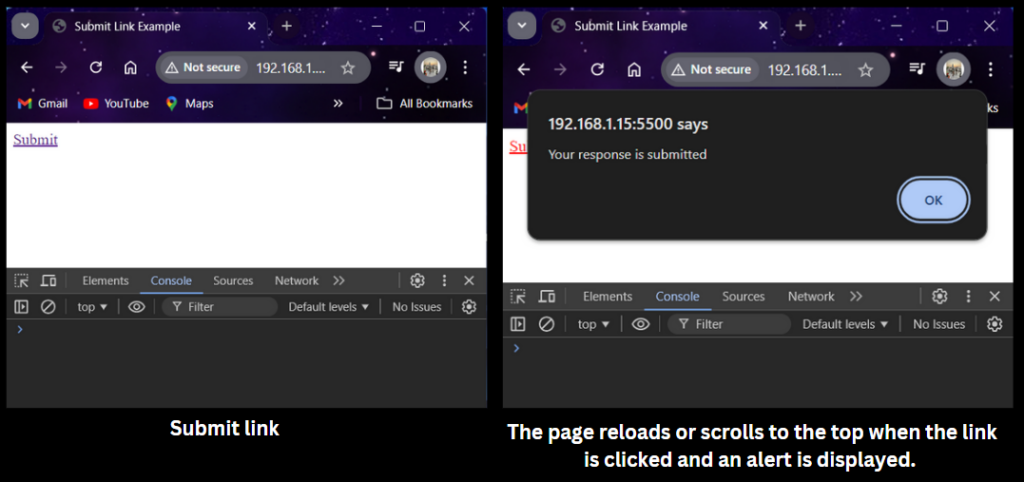
To avoid such problems, developers use javascript:void(0) as the href. This makes the link do nothing by default. It prevents the browser from following the link or refreshing the page but still lets the developer run JavaScript code when the link is clicked.
How Does javascript:void(0) Work
Suppose we wish to add a link to our webpage. Let’s say we are adding a link to our website, i.e., https://codeforgeek.com/. And we want that if the user clicks on the link, an alert is displayed.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Submit Link Example</title>
<script>
function showAlert() {
alert("Welcome to codeforgeek!");
}
</script>
</head>
<body>
<a href="https://codeforgeek.com/" onclick="showAlert()">www.codeforgeek.com</a>
</body>
</html>
This code will surely show the alert after the user clicks the link, but it also redirects us to the linked website.
Output:
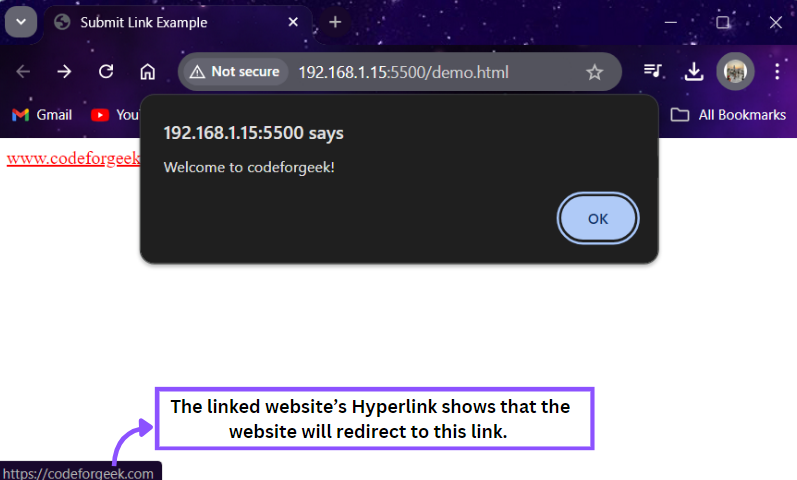
Now suppose we don’t want our webpage to redirect somewhere and prevent it from reloading. To stop this redirecting, we use javascript:void(0) inside the href attribute.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Submit Link Example</title>
<script>
function showAlert() {
alert("Welcome to codeforgeek!");
}
</script>
</head>
<body>
<a href=" javascript:void(0); https://codeforgeek.com/" onclick="showAlert()">www.codeforgeek.com</a>
</body>
</html>
Output:
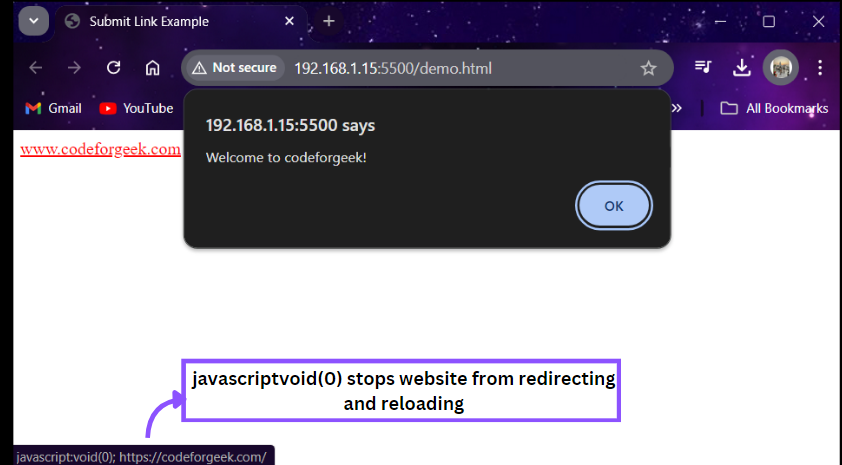
Example of javascript:void(0)
Let’s see another example where javascript:void(0) comes in handy. We will create a Submit link and display a success message on clicking the link without a page refresh.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Show More Example</title>
<style>
#extraContent {
display: none;
margin-top: 10px;
background-color: #f9f9f9;
padding: 10px;
border: 1px solid #ccc;
}
</style>
</head>
<body>
<a href="javascript:void(0)" onclick="document.getElementById('extraContent').style.display='block';">Submit</a>
<div id="extraContent">
Your file has been submitted successfully!
</div>
</body>
</html>
In this code, javascript:void(0) is used in the href to stop the page from reloading or navigating anywhere when the “Submit” link is clicked. Instead, the onclick event is used to display hidden content (#extraContent). This allows JavaScript to run when the link is clicked, without causing any default browser behavior like reloading.
Output:
Conclusion
In this article, we have learned what is javascript:void(0), how it works, and why we use it. The significance of javascript:void(0) is that it prevents the link from doing its primary task, that is, reloading or changing navigations. This becomes important when the developers wish to run some JavaScript through a link click without causing a reload or leaving the page, which would be disruptive to the user.
Do you know what a JavaScript double question mark is? Click here to learn!
Reference
https://stackoverflow.com/questions/1291942/what-does-javascriptvoid0-mean