So you have covered the basics of JavaScript what now? Well with the power of Tensorflow.js, you can now take your JS skills to the next level with Machine Learning. Machine Learning is the use of computer systems that are able to learn and adapt without explicit explanation. Tensorflow.js is a JavaScript library to use on the web.
The prerequisite for using Tensorflow.js is that you should have a basic knowledge of HTML and JavaScript, so without wasting much time let’s get started!
Also Read: What is Node.js?
What is TensorFlow?
A tensor is a mathematical representation of a scalar (a tensor of rank 0), vector (a tensor of rank 1), dyad (a tensor of rank 2), triad (a tensor of rank 3) etc. i,e, a tensor is the generalization of scalars, vectors and the like. And because the idea of any Machine Learning algorithm relies heavily on data usually in the form of scalars, vectors and matrices, this idea of tensors has been chosen by Google. TensorFlow is Google’s open-source Machine Learning library, it is written in C++.
Does that mean we won’t be able to learn Machine Learning if we don’t know JavaScript? Absolutely not! Just like it is easy to use tensorflow.js as a library in JS you can find almost all of the examples in TensorFlow have some sort of bindings to use in Python as well as Java. Tensorflow was open-sourced in 2015 so you can find out pretty much all of the basic examples if you want.
Why TensorFlow?
TensorFlow is developed by Google and is a fantastic Machine Learning Library. But there are also several reasons to check out TensorFlow instead of other ML Libraries: TensorFlow allows us to access device features such as the camera attached or mic attached to our devices. Also using the tensorflow.js library you can integrate Machine Learning into your already existing web pages and web apps that run using Vue, React etc. In fact, you could also import python models to the web using the tensorflow.js library, hence it is a highly flexible library!
Integrate TensorFlow.js into your Project
There are several ways that you can integrate Tensorflow.js into your project! In this article, we will simply use the CDN and integrate the library using a script tag. Here’s the CDN link:
<!-- Load TensorFlow.js from a script tag -->
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs@latest/dist/tf.min.js"></script>
So, simply fire up an HTML file and give the script tag just above the closing head tag and you will have the library loaded. Here’s what the whole code should look like in an index.html file:
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="utf-8" />
<title>TensorFlow.js browser example</title>
<!-- Load TensorFlow.js from a script tag -->
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs@latest/dist/tf.min.js"></script>
</head>
<body>
<h1>My TensorFlow.js Project</h1>
<script>
//We write our tensorflow code here
console.log(tf) //tf is the whole TensorFlow Library, we verify this way that the library has loaded.
</script>
</body>
</html>
Now that we have our TensorFlow library loaded into our project let us learn in the next section how to create tensors!
Creating your First Tensor
In tensorflow.js you can use the tf.tensor() method to create your own tensors. We will however need to run the code inside an async function in order to load the library first. Let us see the following code:
<script>
//We write our tensorflow code here
//console.log(tf) //tf is the whole TensorFlow Library, we verify this way that the library has loaded.
async function run() {
//Write your code here
}
// function to check the DOMContentLoaded criteria,
// we don't want to call the run function too early
if (document.readyState !== "loading") {
run();
} else {
document.addEventListener ("DOMContentLoaded", run);
}
</script>
So we are checking first that the content has loaded and then only we run the code inside the async function! Now to create your own tensors (scalars, vectors or matrices) you can use the tf.tensor() method as follows:
const myTensor = tf.tensor([0, 1, 2]);
console.log(myTensor);
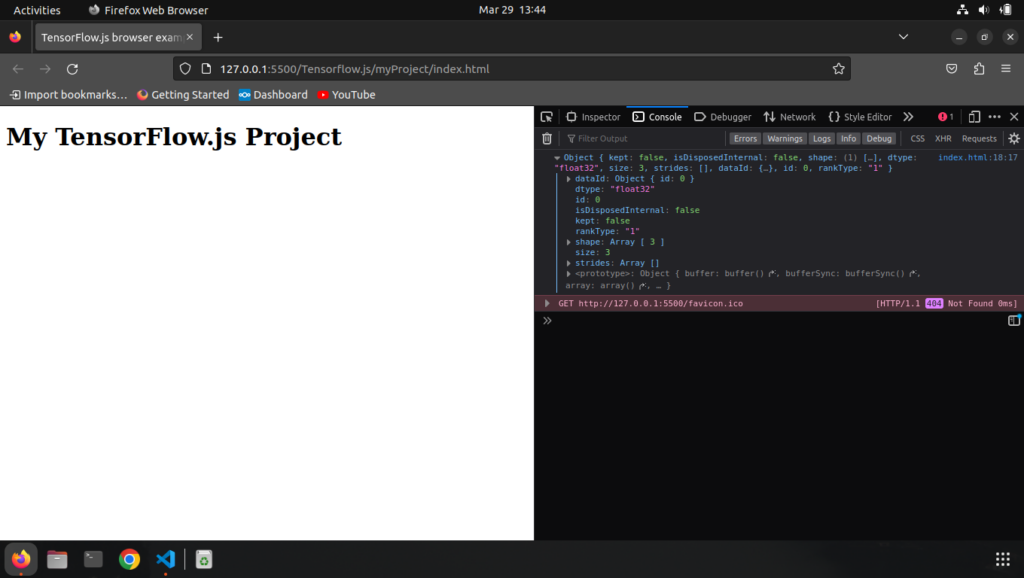
Console logging a tensor is not a good idea, instead, we can use the built-in print() method:
myTensor.print()
Complete Code:
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="utf-8" />
<title>TensorFlow.js browser example</title>
<!-- Load TensorFlow.js from a script tag -->
<script src="https://cdn.jsdelivr.net/npm/@tensorflow/tfjs@latest/dist/tf.min.js"></script>
</head>
<body>
<h1>My TensorFlow.js Project</h1>
<div id="demo"></div>
<script>
//We write our tensorflow code here
//console.log(tf) //tf is the whole TensorFlow Library, we verify this way that the library has loaded.
async function run() {
//Write your code here
const myTensor = tf.tensor([0, 1, 2]);
myTensor.print()
}
// function to check the DOMContentLoaded criteria,
// we don't want to call the run function too early
if (document.readyState !== "loading") {
run();
} else {
document.addEventListener("DOMContentLoaded", run);
}
</script>
</body>
</html>
Output:
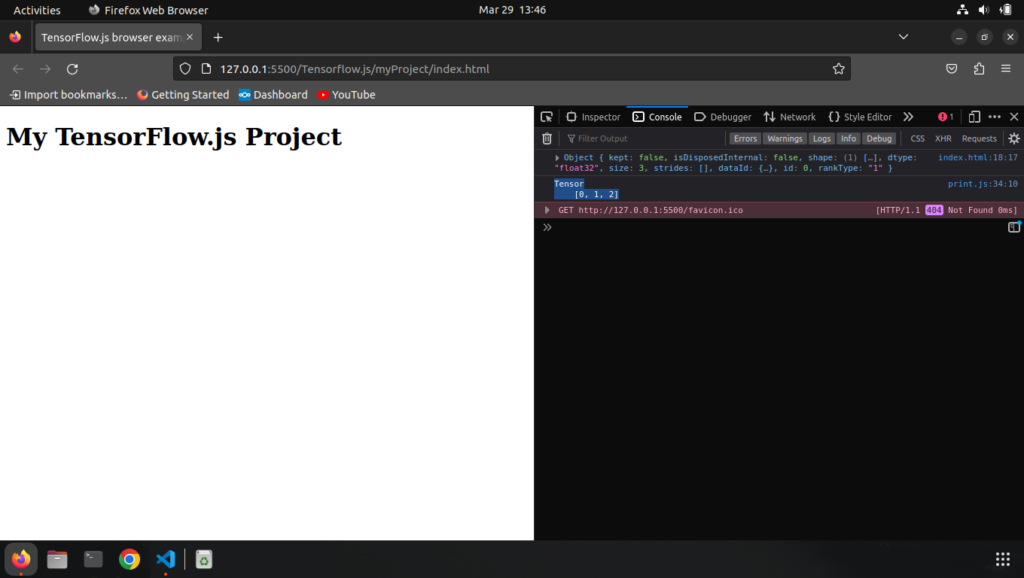
Conclusion
In this tutorial, we’ve covered an introduction to TensorFlow.js, various concepts in TensorFlow.js, and creating a Tensor. We have used native HTML and JS code to make the concept as simple as possible. Hope this tutorial helped.
Reference
https://www.tensorflow.org/js/tutorials