How to generate a random number in Solidity – is the question that brought you here today. Fortunately, you are at the right place!
Since Solidity, Blockchain, Ethereum, and all other things related to these are relatively new for learners, it becomes difficult to understand what is going on sometimes and why.
The Solidity programming language is also very much new when compared to other programming languages out there in the wild.
There are many languages in the programming industry, some of which we as developers may have never heard of. The reason for this is that large-scale breakthroughs occur on a regular basis, as well as the emergence of additional programming languages. The Solidity programming language is only one of the many amazing languages available.
What Is Solidity In A Nutshell?
Solidity is an object-oriented programming language designed to build smart contracts on the Ethereum network, or the Ethereum Virtual Machine (EVM). It is a curly brace language, according to the official description.
Solidity will be better to comprehend if you are acquainted with Python, C++, or JavaScript because it is related to those languages. This language, like most others, allows inheritance, libraries, tools, and complicated user-defined types, which makes writing easier for programmers.
The ‘.sol’ file extension is used to identify Solidity files. It’s a statically typed programming language. Solidity v0.8.9 is the most recent stable version as of this writing. Click here for a link to the official Solidity documentation.
Let us now move on to our guide to generate a random number in Solidity.
How to Generate a Random Number in Solidity?
Let’s face it, unlike other programming languages like JavaScript, you do not get a native function that allows you to create or generate a random number in Solidity. To accomplish something like this, we go by a way called pseudo-random number generator in Solidity.
A pseudo-random number generator is called by that name because it doesn’t really take random values to it – obviously because there is no room for that here in Solidity. We must ourselves create a pseudo-random number generator by creating a function.
This pseudo-random generator takes in things that we specify, whose values are known to us ahead of time somehow. This random value generated is a hexadecimal hash which we pass into the keccak256 algorithm.
The keccak256 function simply converts the value into a hash value. We will take the hash value and then parse it to translate it into an unsigned integer value or uint.
Now, let us take a look at an example smart contract code that I will write in the Remix IDE to explain clearly how to generate a random number in Solidity using keccak256.
Creating a Smart Contract to Generate a Random Number in Solidity Using keccak256
Let us say we want to create a raffle smart contract where there is a raffle organizer (organizer) that is indicated by the address of the organizer. There are the addresses of the entrants for the raffle (players).
We shall then be able to enter into the raffle and all the Ether that we collect in the prize pool should be transferred to the winner. We will also add more other logic into our smart contract.
So, let us get started:
- To generate a random number in Solidity, we are creating a smart contract. We will write our smart contract in the Remix IDE for now and deploy our contract on the blockchain.
- In the Remix IDE, on the left sidebar, look for a contracts folder and click it
- Look for a file called Ballot.sol and open it up. Clear out all of the code that is there. If you have created some contract and need for future use, please save it on your machine for now.
- Or, you can simply create a new file in the contracts folder.
- I am going to write Solidity 0.4.17, so please make sure the compiler is of the same version too. To change the compiler version, simply click the Solidity or S icon on the left-most thin sidebar. Set the compiler version to 0.4.17+commit.bdeb9e52.
- Make sure you have checked the auto-compile checkbox
- Now flip over to your code editor and create a smart contract.
The Raffle Smart Contract Code to Generate a Random Number in Solidity
Here is what my code looks like to generate a random number in Solidity:
pragma solidity ^0.4.17;
contract Raffle {
address public organizer;
address[] public players;
function Raffle() public{
organizer = msg.sender;
}
function enter() public payable{
require(msg.value > .01 ether);
players.push(msg.sender);
}
function random() private view returns(uint){
return uint(keccak256(block.difficulty, now, players));
}
function pickWinner() public{
uint index = random() % players.length;
players[index].transfer(this.balance);
}
}
Take a look at the random() function and the pickWinner() function.
The random() simply takes the difficulty of the block to get mined which is denoted by a number, the current time, and the addresses of the players. It then takes all of these and passes them into the keccak256 algorithm which is an instance on the sha3 class of algorithms.
All of this is then passed into the uint function to convert the returned value into an unsigned integer.
From there, the pickWinner() function takes the value of the random() function and mods it with the length of the players array. This eventually does generate a random number in Solidity with the help of our Pseudo-random number generator.
Testing the Raffle Contract Using Remix
Let us now deploy and test our contract to check if it is working.
Deploying the Contract for Testing in Remix
Start with the steps below:
- First, make sure you have set the compiler version to 0.4.17+commit.bdeb9e52 in the remix editor. If not, click on the Solidity Compiler tab (Solidity logo), on the farthest left icon menu and do so under the ‘Compiler’ dropdown. Also, make sure you have checked the auto-compile button.
- Next, click on the Ethereum with arrow icon to deploy and run our contract.
- Make sure you do not have any deployed contracts. If you do click the trash icon besides the Deployed Contracts heading to delete all existing contracts.
- Now, hit the Deploy button.
- You will now see a deployed contract with the name Raffle, under the Deployed Contracts section.
- To make sure your contract was deployed successfully open the console to see a success message like this:

Testing Functions of the Contract in Remix
Let us now test all the existing functions in our contract.
- Pull open the deployed contract by clicking on the down arrow button beside it.
- You should now see a list of functions that are available for us to call, like this:
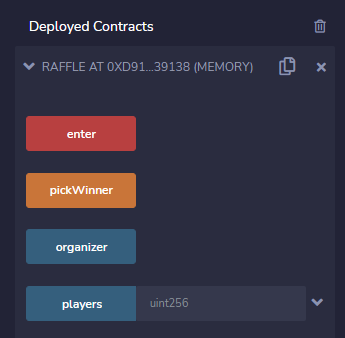
- Let us start with the enter function. Simply click the enter function to enter the raffle. We can even choose a different account to enter, which is what I will do.
- To change the account and enter, scroll to the tab inside the deploy tab and look for the Accounts tab. Choose from list of accounts. You do not want to use same account you used to deploy.
- Next, enter the amount of ether you want to put in. Remember, we set a minimum of 0.01 Ether for entry. Also, make sure you select ether as the token used for the transaction. By default, wei is selected.
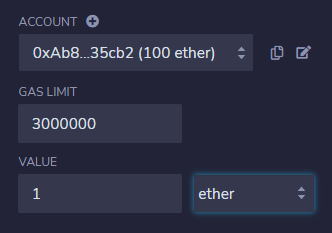
- Now, scroll down below until you the enter function, and click it. Make sure your console prints a success message like this:

- Next, let us check if we were entered into the raffle. We will call the players array and ask it give us the value at index 0, which is the first value. You should see the account address printed of the account you used to call the enter function.
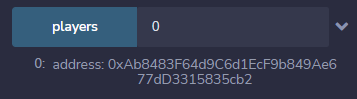
- We can also call the organizer function to see who has organized this raffle contract.
- Finally, we can test out the pickWinner function. If you want you can enter using other of your accounts to have a nice list of players to choose a winner from. I have done the same and now have a good amount of prize money.
- Click the pickWinner function and look for a success message in the console:

- Now, all the balance remaining in the contract, or the prize money so to say, we will be transferred to a winner. In my case it is the first player who entered. Great!
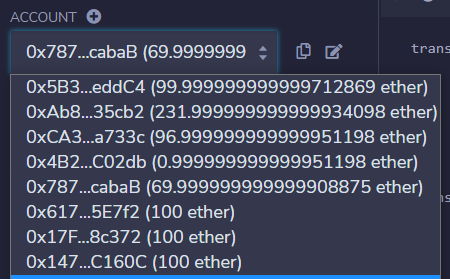
Congrats 0xAb8…35cb2 on winning the raffle!
Read More: How to Use Solidity on VS Code
Conclusion
Learn to generate a random number in Solidity with keccak256.