Desktop notification is a way to notify user about important events even if the browser or tab is inactive or minimized. This increase the user experience and also keeps the user engage with your applications.
In this tutorial i am going to show you how to develop Desktop notification system using core HTML5 and JavaScript.
Some famous applications already using Desktop notification like Gmail using it to notify new email received. Facebook use it for notifying user about any activity such as if any one commented on your post it or like it.
Note : Facebook have Desktop notification as well as internal notification system which works in the facebook tab only. Don’t mix both of them, they are different.
Design:
I have developed a Desktop notification which look like this. In this we have option to put icon, title and body text.
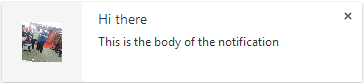
Code:
Creating a Desktop notification is as easy as the line of code shown below.
You can pass arguments to Notification function and they are as follows:
- title: Title of notification
- body: Content of Notification.
- icon: Icon path (URL,image or blank).
- tag : ID of notification.
- dir: Direction of Text. (left,right,auto)
Complete JavaScript code:
function notifyMe() {
if (!("Notification" in window)) {
alert("This browser does not support desktop notification");
}
else if (Notification.permission === "granted") {
var options = {
body: "This is the body of the notification",
icon: "icon.jpg",
dir : "ltr"
};
var notification = new Notification("Hi there",options);
}
else if (Notification.permission !== 'denied') {
Notification.requestPermission(function (permission) {
if (!('permission' in Notification)) {
Notification.permission = permission;
}
if (permission === "granted") {
var options = {
body: "This is the body of the notification",
icon: "icon.jpg",
dir : "ltr"
};
var notification = new Notification("Hi there",options);
}
});
}
}
</script>
To trigger a Desktop notification, here is a HTML code. Put this under <body> tag.
Explanation:
if you look over the JavaScript code then you might notice that code runs after doing some important validation. Those validations are performed in order to ensure that application using Desktop notification runs under the sandbox defined by browser.
Step 1: Checking whether browser support Desktop notification or not.
Step 2: Checking if permission is already granted (means it is not a first visit.), if it is then show the notification.
Step 3: Checking if permission is not “denied” by user externally, then requesting browser to confirm the permission.
if (!('permission' in Notification)) {
Notification.permission = permission;
}
Step 4: If user allow the permission then we will show the notification else not.
Running the code:
You can download the code by provided link and make sure you run it by Web Server. If you will directly run it like static HTML then you will probably not get the output. Place it in HTDOCS folder and access it via URL.
Once you will click on the button, Browser will ask you for permission. Allow it and then see the output on bottom-right of Desktop.

Further Reading:
Example above is static. You have to click a button and it will show you the notification. Real time application need more than this. So let’s discuss one real time example with Desktop notification.
For example you are building an email reader app by having Node as backend and angular as front-end. You have timer job running which checks for arrival of new emails in database. You can use Notification in real time app like the one shown below.
.controller('email_control',function($scope,$interval,$http){
var timer=$interval(function(){
$http.post(“API to check new emails”,function(data){
//just assuming that data consist of every parameter we used below.
//Also desktop notification is enabled in setting of app.
if(data.new==1)
{
var options = {
body: data.email.excerpt,
icon: "icon.jpg",
dir : "ltr"
};
var notification = new Notification(data.email.title,options);
//onclick do some action, like redirect or new window
notification.onclick=function(){
};
//on display of notification do some action like play sound
notification.onshow=function(){
};
}
});
},100);
});
You can see the complete specification here.