API stands for Application Programming Interface that acts as a bridge between two systems such as a client and server in order to perform various operations on data.
The client i.e the system which uses API can request different data from the server which contains the API logic with the database. Using the API, the client can not only get the data but also create new data records, update pre-existing data and delete the data records.
On the other hand, when we are creating the API, we create it in a way that it can perform various operations on the database.
A CRUD API is an API that can able to perform the CRUD operation which stands for Create, Read, Update and Delete.
- Create: New records can be created in the database.
- Read: The record can be read.
- Update: The API can able to update the pre-stored record.
- Delete: The API can able to delete the selected records.
Build a CRUD API in Node.js
Let’s create a CRUD API that creates new book record, read all records, update records and delete records.
Create a folder containing a file “app.js” & open it inside the Code Editor.
Then in the terminal, type the following commands
npm init -y
This will initialize the Node Packages manager, by which we can install any Node.js framework.
npm i express
this will install express as a module in your application.
Inside the “app.js” file, import the express module.
const express = require("express");
Create a constant “app” and set it to “express()”, which means creating an instance of an express module, by which any of the express functions can be called. Then use the express middleware to parse JSON data in order to fetch data from the client.
const app = express();
app.use(express.json());
app.use(express.urlencoded({extended: true}));
Create an array that can hold the book record.
const books = [
{
bookId: "1",
bookName: "The Rudest Book Ever",
bookAuthor: "Shwetabh Gangwar",
}
];
Then use the express get method to define the logic for the “/” route. Inside this method, use res.send() method to send the books array we just created as a response.
app.get("/", function (req, res) {
res.send(books);
});
Use the post method of express to handle the post request, where we fetch the data that comes from the client and push them into the book array we created earlier.
app.post("/", (req, res) => {
const inputbookId = books.length + 1;
const inputbookName = req.body.bookName;
const inputbookAuthor = req.body.bookAuthor;
books.push({
bookId: inputbookId,
bookName: inputbookName,
bookAuthor: inputbookAuthor
});
res.send("Book Added Successfully");
});
Again, use the post method, this time the route is the delete route which is used to delete a record whose Id is fetched from the client.
app.post("/delete", (req, res) => {
var requestedbookId = req.body.bookId;
var j = 1;
books.forEach((book) => {
if (book.bookId === requestedbookId) {
books.splice(j-1, 1);
}
j = j + 1;
});
res.send("Deleted");
});
Then finally create the route for updating the book record, where we fetch the book on the basis of Id and then update the records whose key and value are fetched from the client.
app.post("/update", (req, res) => {
const requestedbookId = req.body.bookId;
const inputbookName = req.body.bookName;
const inputbookAuthor = req.body.bookAuthor;
var j = 1;
books.forEach((book) => {
if (book.bookId == requestedbookId) {
if(inputbookName){
book.bookName = inputbookName;
}
if(inputbookAuthor){
book.bookAuthor = inputbookAuthor;
}
}
j = j + 1;
});
res.send("Updated");
});
Create a port to test our application, using listen() method, and pass the 3000 port as an argument.
app.listen(3000);
Now, inside the postman search bar and type the below URL and perform the different operations to see the working of the API.
http://localhost:3000/
Complete Code:
const express = require("express");
const app = express();
app.use(express.json());
app.use(express.urlencoded({extended: true}));
const books = [
{
bookId: "1",
bookName: "The Rudest Book Ever",
bookAuthor: "Shwetabh Gangwar",
}
];
app.get("/", function (req, res) {
res.send(books);
});
app.post("/", (req, res) => {
const inputbookId = books.length + 1;
const inputbookName = req.body.bookName;
const inputbookAuthor = req.body.bookAuthor;
books.push({
bookId: inputbookId,
bookName: inputbookName,
bookAuthor: inputbookAuthor
});
res.send("Book Added Successfully");
});
app.post("/delete", (req, res) => {
var requestedbookId = req.body.bookId;
var j = 1;
books.forEach((book) => {
if (book.bookId === requestedbookId) {
books.splice(j-1, 1);
}
j = j + 1;
});
res.send("Deleted");
});
app.post("/update", (req, res) => {
const requestedbookId = req.body.bookId;
const inputbookName = req.body.bookName;
const inputbookAuthor = req.body.bookAuthor;
var j = 1;
books.forEach((book) => {
if (book.bookId == requestedbookId) {
if(inputbookName){
book.bookName = inputbookName;
}
if(inputbookAuthor){
book.bookAuthor = inputbookAuthor;
}
}
j = j + 1;
});
res.send("Updated");
});
app.listen(3000);
Output:
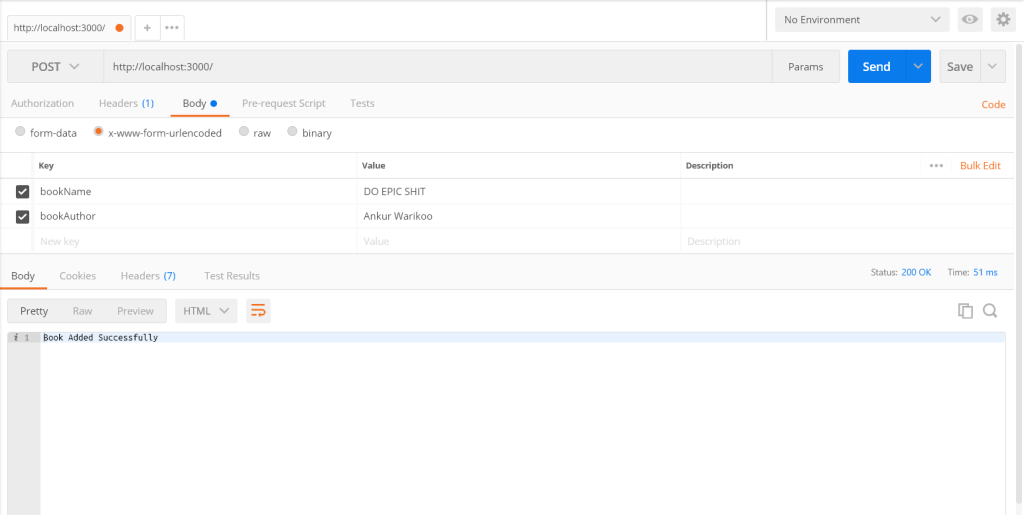
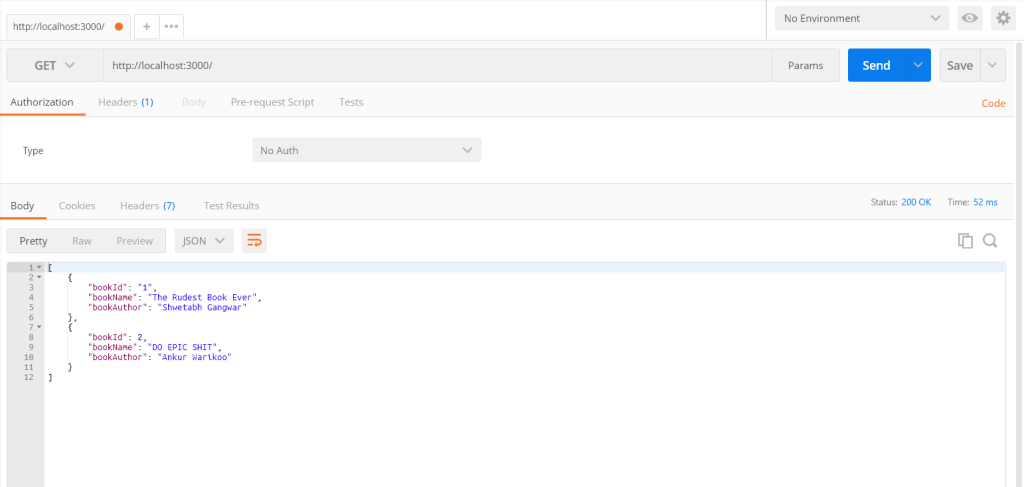
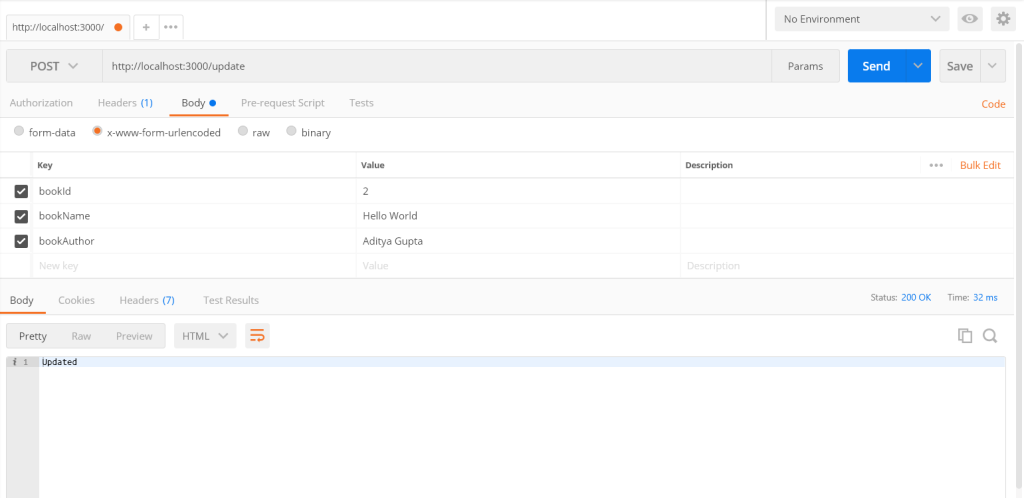
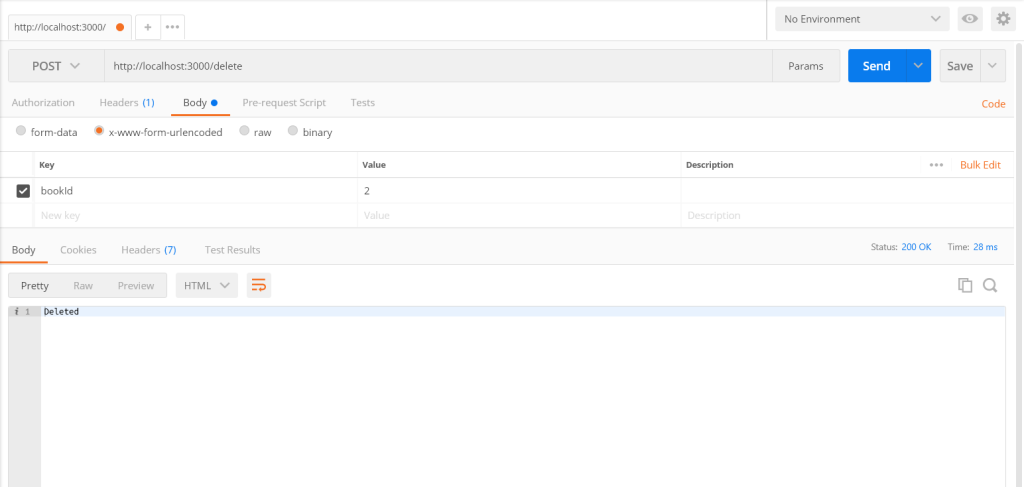
Summary
A CRUD API is used to interact with the server data to perform create, read, update and delete operations with the database record. CRUD API gives the power to interact with the server directly using different GUI Interface applications such as Postman. We hope this tutorial helps you to learn the mechanism of creating a CRUD API in Node.js.
Reference
https://stackoverflow.com/questions/14990544/how-to-best-create-a-restful-api-in-node-js