One potential use of our coding expertise is in the video game industry. Here, we’ll break down the steps involved in coding a blackjack app. Blackjack’s logic is straightforward, but it’s still complex enough in a way that it’ll help us when we move on to more intricate games.
The Rules Of Blackjack
Before successfully coding a blackjack app that would serve players amazing gameplay and go on to be a well-known app amongst gamblers, such as those found on Basketball Insiders list of the best real money blackjack apps, one must understand the basic rules of the card game, and they are as follows:
- Two cards are dealt to each player and the dealer from a shuffled deck. Although casinos often utilize a “shoe” made up of six decks, we’ll just use one in our case.
- After the dealer and player get their first two cards, the player is asked whether they want another (called “hitting”) or if they are satisfied (called “standing”). Get your card values as close as 21 without going over. If we hit 21, we have unbeatable blackjack. Over 21 “busts” the round. Any player may cease hitting.
- Face cards are worth 10, number cards (2–10) have their specified value, and an ace might be 1 or 11. If we were handed a Jack and an Ace as our first two cards, we’d count the Ace as 11 to get blackjack. If we hit after obtaining a hand valued 18, we would consider the Ace as 1 since going with the latter would make us bust at 29.
- The dealer attempts to follow suit when we have finished playing our hand. The dealer must continue to strike until they reach 17. They may continue if they reach the 17 without busting.
First Steps For Coding Blackjack Apps
So, first things first, we must code a shuffled deck of cards in order to kick off our game of blackjack.
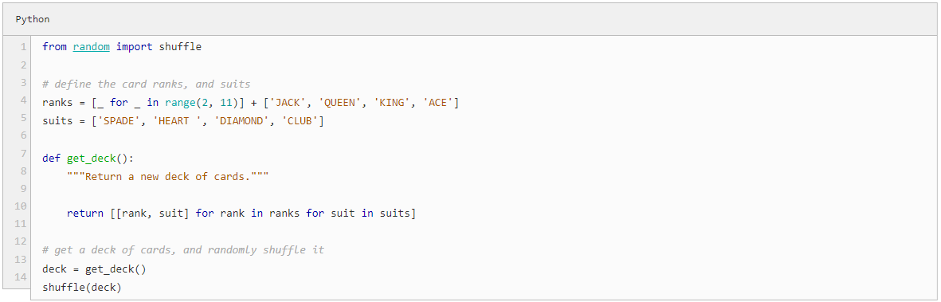
We used lists to organize the card suites and ranks, and then we created the straightforward method get_deck(), which builds the deck using a list analysis.
Finally, we utilized the random library in Python, which offers a number of methods for producing unpredictability. We specifically use shuffle to shuffle our deck of cards, which accepts any list and returns it in random order.
We may deal the first two cards to the player and the dealer once this functionality is in place. Likewise, we use the destructive pop operation, which removes the last member from a list as a byproduct of returning it. Assuming the player hasn’t busted and hasn’t requested to “stand” with their present hand, we additionally establish a boolean variable to monitor whether they are still actively betting.

The Scoring Function
Next, we create a function that receives a list of tuples representing the hand’s cards (rank, suit). The reasoning is rather basic. The first thing we do is create a helper function that accepts a single card and returns its value using the above-described method. We automatically count each ACE as 11. The total of the values is stored as tmp_value once this function is mapped across the hand.
Adding up the total number of aces in the hand is the second major point. The value of the hand is next checked to verify whether it is less than or equal to 21. If it isn’t and there was an ace in the hand, we deduct 11 points. If the hand’s value is below 21, we give it back. Otherwise, we again deduct 11 and check again whether there is a second ace in the hand. We keep doing this until we have used up all the aces in the hand.
Returning the hand’s value is the last step. If the score is less than 21, we provide a two-entry list with the integer score value and a string representation of the score. Busts are counted as one hundred by tradition.
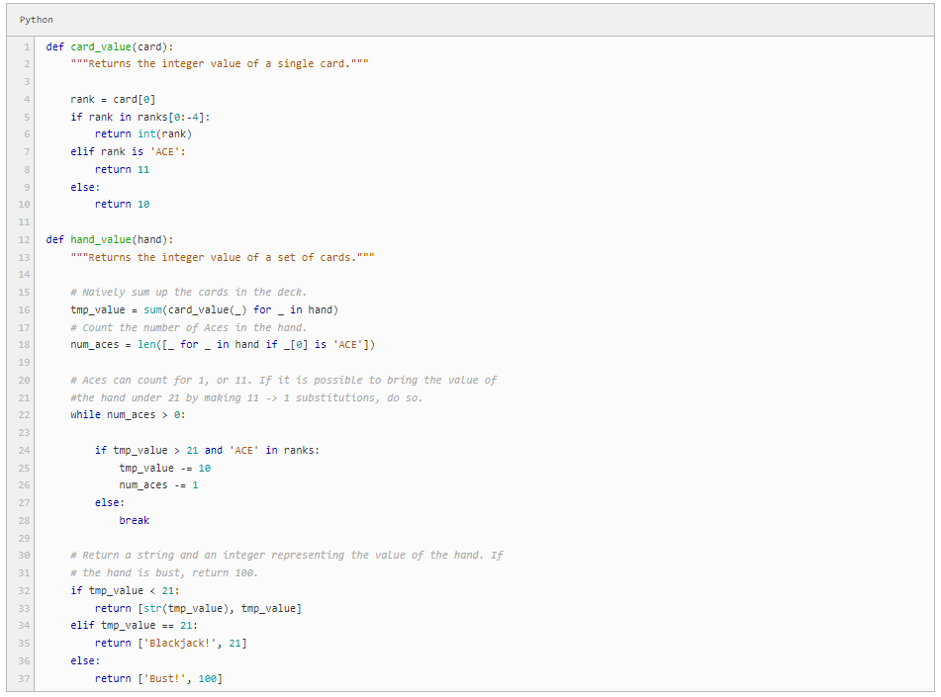
Game Logic
The gaming logic is the next element we code. As previously said, we must ask the player whether they want to hit or stand. The player’s current tally is one piece of information that is essential to their choice, therefore we publish the player’s hand and tally before requesting their answer.
We use Python’s raw_input function, which accepts keyboard input, to pose the query as long as the player’s hand doesn’t turn out to be a bust. We use 1 to represent “hit me” and 0 to represent “stand” for convenience. If they request a hit, we once again pop the deck, add the new card to our hand, and then print the freshly drawn card on the screen. We set player_in to False and go on to the dealer.
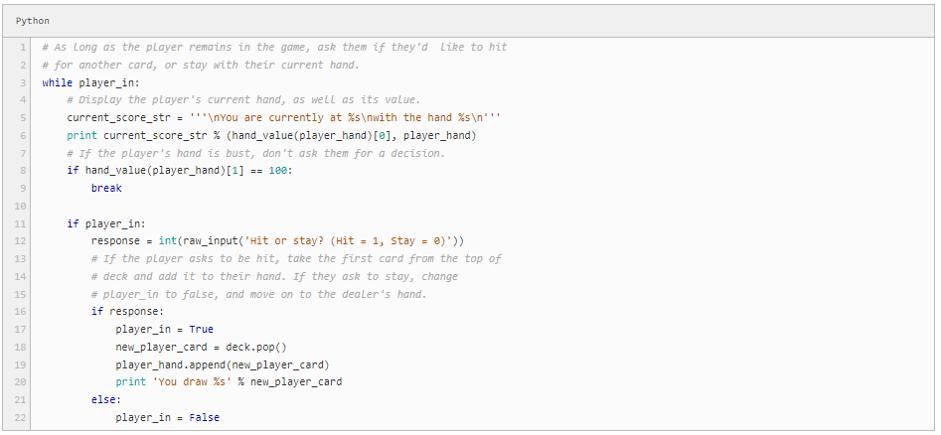
We now determine the player’s score as well as the dealer’s current score (on his first two cards). We print the dealer’s score and the player’s hand if the player’s hand isn’t busted. The dealer is therefore forced to hit even if his hand is valued less than 17. We print the dealer’s fresh card after each hit. This loop is intended to end when the dealer reaches 17.
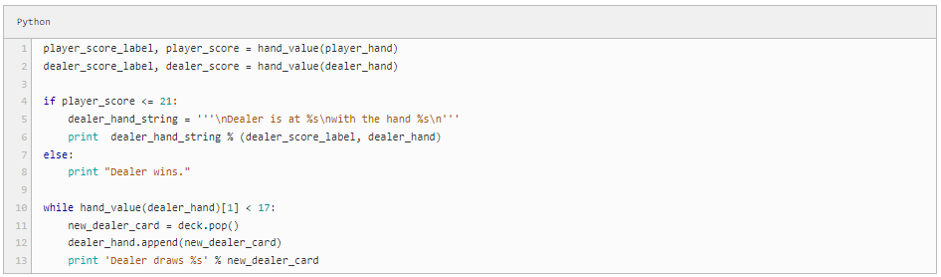
Setting The Winner
Almost done now! Check the player’s and dealer’s scores against the aforementioned scoring guidelines. First, we get the dealer’s label and score. Next, we list alternative end states and ask which one of them our game meets. Depending on the result, we publish the winner.
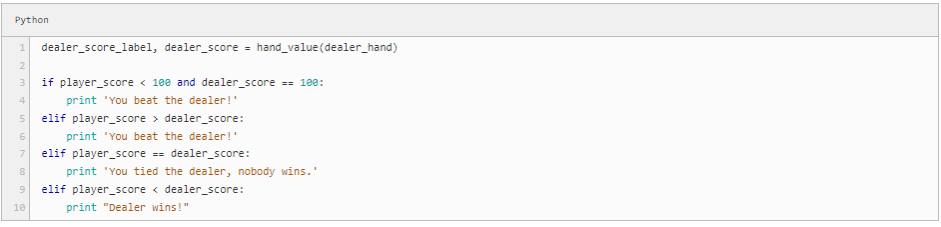
Additionally, just as websites use reCAPTCHA to secure their services, you may want to look into a form of security for your app.
Sample Gameplay
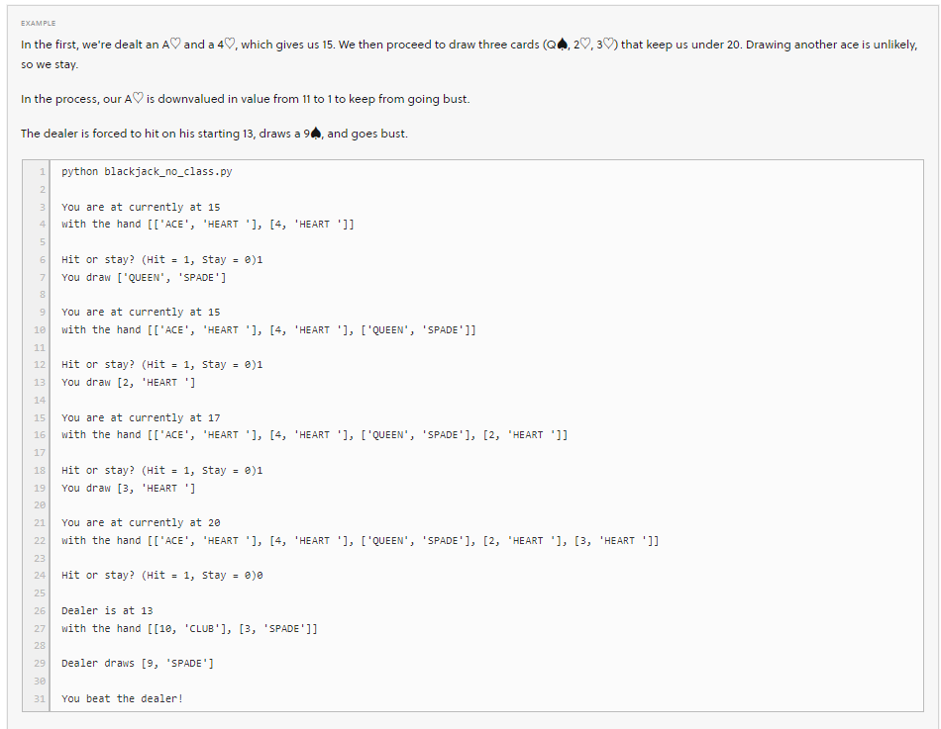
Alternatively, there’s the case where the dealer wins, and we go bust.
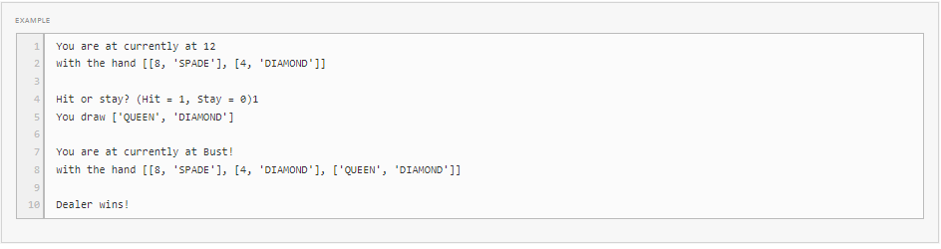