NodeJS Debugger is a tool accessed through the command line that helps to debug the Node.js code. It provides a way to set different breakpoints to stop the program’s execution at a point to find the bug. Node.js Debugger is also efficient to track the value of variables that how they change with the flow of execution.
Also read: NodeJS Crypto Module – Encrypt and Decrypt Data
Node.js is mainly used to create servers, APIs, and web services, which can be heavily coded, with complex structures and it is hard to debug it. It is very overwhelming for a developer to find which line exactly produces the bug.
The simple solution is Node.js Debugger which provides various tools to debug the code using the terminal.
Node.js Debugger
For using Node.js Debugger it is essential that you have Node.js installed on your pc. For initiating the Node.js Debugger use the below command.
Syntax:
node inspect file_name
Where file_name is the name of the file you want to inspect.
Node.js Debugger Commands
Node.js Debugger provides various commands which are important to learn before understanding the working of Debugger.
- c/cont – This command is used to execute the code till a breakpoint or end.
- n/next – This command will move the execute to the next line.
- s/step – Use to get inside of a function.
- o – Use to get out of a function.
- pause – pause the execution.
These commands might be difficult for you to understand, but soon we will see a fully working example that will clear your doubt.
Working of Debugger
For understanding the working of Debugger, let’s create an example project containing a single file app.js with the following code.
let numbers = [1, 2, 3, 4, 5];
let sumOfNumbers = 0;
for (let i = 0; i <= numbers.length; i++) {
sumOfNumbers += numbers[i];
}
console.log(sumOfNumbers);
To run the application, locate it inside the terminal and type the following command
node app.js
The output we expected is 15.
The output we get is:
NaN
Now, we will use Debugger to find the bug in the program.
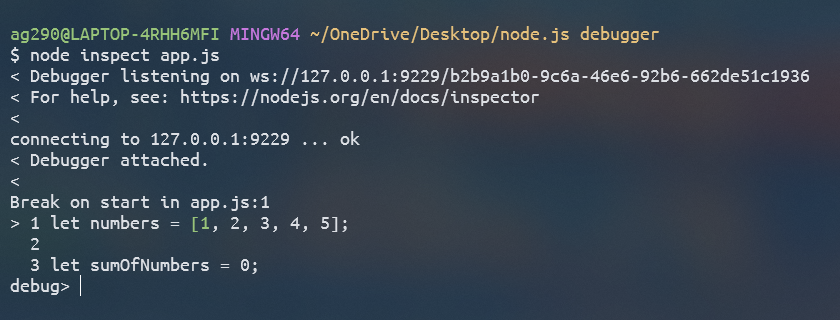
We see that the execution starts from the top and as we press n it will move to the next line.
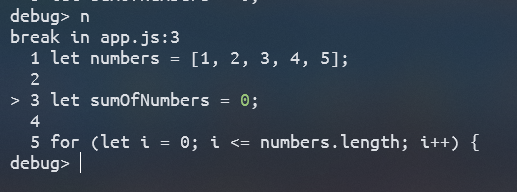
Now we have to use the watch method to track the variable, i, and sumOfNumbers. This method takes an argument, a string containing the name of the variable to track its value.
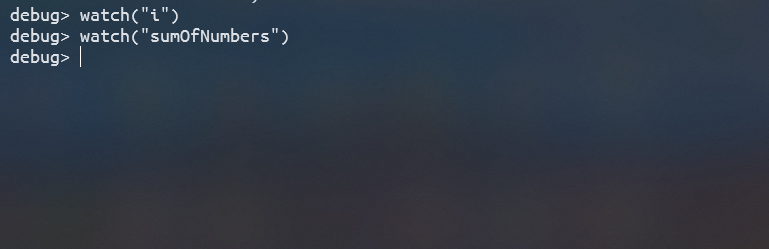
As we proceed we see that the value of both variables changes as expected, in each loop the value of the array’s next element is added to the sumOfNumbers variable.
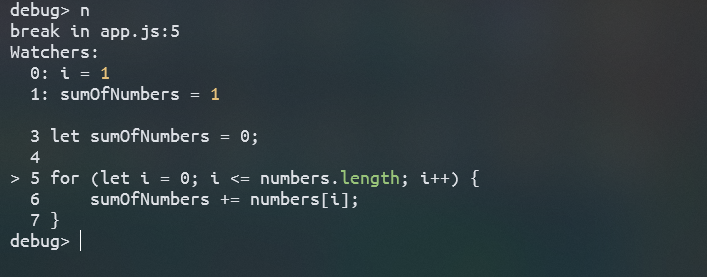
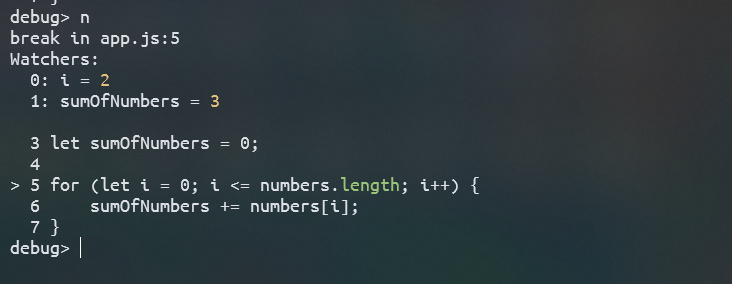
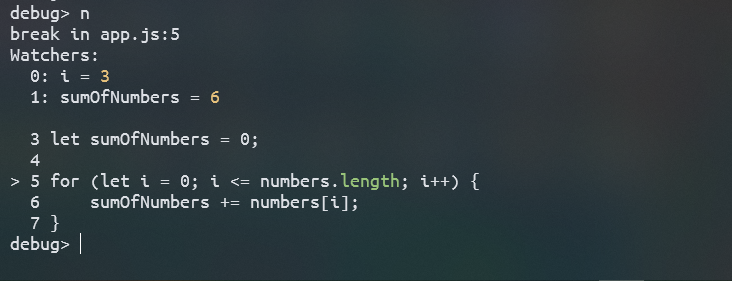
Let’s run the loop until the value of sumOfNumbers becomes NaN.
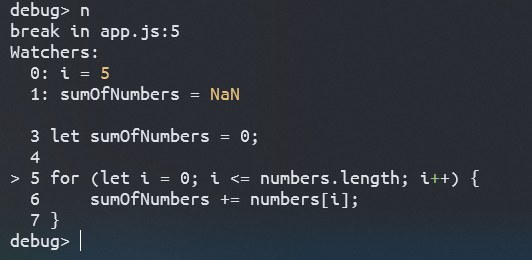
Now, we see that the bug happens when the value of variable i becomes 5, and we notice that the array has only 5 elements since the elements of an array start with index 0, so the last element of the array should be at index 4, but our value of i becomes 5 and it tries to add the value of the element in the index 5, but no element exists at that index so the default value of the element at index 5 is NaN, that’s why the sumOfNumbers becomes NaN.
From the above observation, we conclude that the value of i should not be greater than 4 as it is the last index of the array, so we just remove the = symbol in the for loop and run the code again.
Output
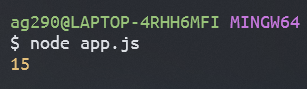
We get the same output as expected.
Summary
Node.js Debugger helps to debug the code directly by using the command line interface, it comes with Node.js, so for using it Node.js should be installed. You can go through the line-by-line execution of the code to find the bug using the debugger. Hope this article helps you to understand the concept of Node.js Debugger.
Reference
https://nodejs.org/api/debugger.html#debugger