If you own or operate a company, you’ve probably had to deal with the hassle of validating value-added tax (VAT) identification numbers (IDs) for your international customers. It’s a necessary step to ensure you’re correctly charging customers from different countries, but it’s a manual process that can waste valuable resources.
Fortunately, there is a way to automate the VAT validation process, which can save you valuable time and resources. In this article, we will show you how to create a VAT verification web app using JavaScript.
What is a VAT validation API?
A VAT validation API enables businesses to automate the verification process of VAT numbers. This is important for businesses that deal with multiple VAT-registered companies in different countries, as it can save time and avoid mistakes in the verification process. The API checks the number against government databases in the EU, Australia, Norway, Switzerland, and the UK to see if it’s real and up-to-date and if the correct tax treatment has been applied.
This process is important for businesses because it helps them ensure they comply with regulations and limits the risk of fines or penalties. It’s also useful for customers, as it gives them confidence that the company they’re doing business with is legitimate.
A Step-by-step on how to create a vat validation web app using JavaScript
In this tutorial, we are going to build a Vat Validation web app using JavaScript, Tailwind CSS, and Vite.
Before we begin, make sure your development stack consists of the following:
- Yarn
- Vite
- Tailwind CSS
- postcss
- autoprefixer
- Vatcheckapi Account (free)
- Vanilla JavaScript
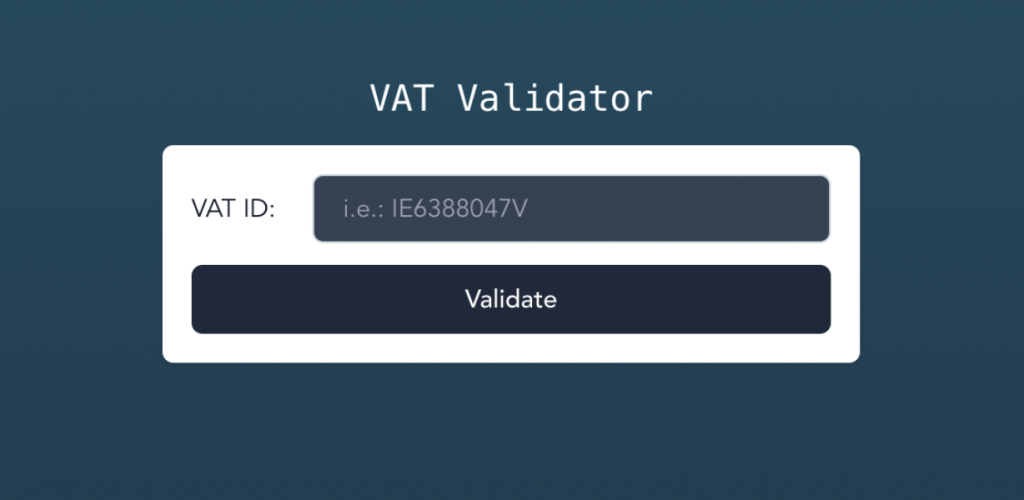
Step 1: Initializing a Vite Project
Our first step is to initialize a Vite project in our development workspace. Vite is a build tool that helps developers create high-quality web applications. It offers a fantastic developer experience and can help to improve the performance of your web app.
We enter: yarn create vite
Once your project is initialized, will now ask us for a project name. After choosing whatever name we want, we need to select a framework. Since we want to use plain JavaScript, we select vanilla (twice).
Next, we switch into the newly created directory, as suggested by the console, but we don’t start the yarn build just yet.
Step 2: Styling setup (optional)
This step is optional, however, if you do decide to follow it, we recommend using Tailwind CSS. Tailwind is a great tool for rapidly prototyping and building out custom designs. It’s also easy to use and get started with. We use it combined with autoprefixer & postcss for a smooth development experience.
To style our validator, we need to install the following packages first:
yarn add -D tailwindcss postcss autoprefixer
Next, we initialize Tailwind. A config file (tailwind.config.cjs) will be created:
npx tailwindcss init
Now, we need to adapt this newly created config to work with our Vite project setup:
/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
'./main.js',
'./index.html',
],
theme: {
extend: {},
},
plugins: [],
}
At the top of our style.css, we need to add the following to include Tailwind CSS:
@tailwind base;
@tailwind components;
@tailwind utilities;
For postcss, we need to create a config file named postcss.config.cjs in our root directory and add the following lines:
module.exports = {
plugins: [
require('tailwindcss'),
require('autoprefixer')
]
}
Step 3: Start vite in dev mode
We’re now ready to start vite to serve our files with hot reloading. To do this, we’ll run the following command:
yarn dev
This command will open a new tab in your browser automatically. If not, open http://localhost:5173/ in a browser of your choice.
You should now see the default Vite index page reading Hello Vite!
Step 4: Prepare your HTML
The next step is to prepare our HTML and adapt the default landing page. We create an index.html file and build a form for the VAT validator. We will need the following:
- A wrapper form id=”vat_id_validator” to hold our input
- An input id=”vat_id” for the actual phone number
- A submit button type=”submit”
- A container div id=”result” where we display our response from the API
Here is what our implementation of the index.html looks like:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8"/>
<meta name="viewport" content="width=device-width, initial-scale=1.0"/>
<title>VAT Validator</title>
</head>
<body class="flex flex-col items-center justify-center bg-gradient-to-b from-cyan-800 to-slate-800 min-h-screen py-5 text-slate-900">
<form
id="vat_id_validator"
class="relative mx-auto w-full max-w-sm bg-white shadow rounded-md p-4 text-sm"
>
<h1 class="absolute text-center left-0 right-0 -top-10 text-xl text-white font-mono">VAT Validator</h1>
<div class="space-y-3">
<div class="flex items-center space-x-5">
<label for="vat_id">VAT ID:</label>
<input
type="tel"
id="vat_id"
name="vat_id"
placeholder="i.e.: IE6388047V"
value=""
class="grow border-slate-300 border rounded-md py-2 px-4 text-sm bg-slate-700 text-white"
required
/>
</div>
<button
type="submit"
class="bg-slate-800 text-white rounded-md py-2 px-4 mx-auto relative block w-full"
>Validate</button>
</div>
</form>
<div
id="result"
class="mx-auto my-5 w-full max-w-sm bg-white shadow rounded-md relative overflow-hidden text-sm empty:hidden divide-y divide-dotted divide-slate-300"
></div>
<script type="module" src="/main.js"></script>
</body>
</html>
Note: You can use any HTML or CSS framework you like, however in this tutorial all CSS classes are tailwind specific, so if you want to use another CSS framework or style it yourself, remove them completely.
The id attributes in JavaScript are used as selectors. If you adapt them in HTML, also adapt them in your JavaScript accordingly.
Step 5: Handle form submission in JavaScript
In our main.js, we read the data from the input and send it to the vatcheckapi. For this to work, we need an API key. In this tutorial, we will be using Vatcheckapi.com, which provides a free API key and a great developer experience.
The main.js could look something like this:
import './style.css'
const vatValidator = document.getElementById('vat_id_validator');
const vatInput = document.getElementById('vat_id');
const resultContainer = document.getElementById('result');
vatValidator.addEventListener('submit', (e) => {
e.preventDefault();
fetch(`https://api.vatcheckapi.com/v2/check/?vat_number=${vatInput.value}`, {
headers: {
apikey: 'YOUR-APIKEY'
}
})
.then(response => response.json())
.then(data => {
if (data.message) {
resultContainer.innerHTML = `<div class="px-5 py-3 border-2 rounded-md border-red-500 text-600 px-5 py-3">${data.message}</div>`
} else {
const borderClasses = data.checksum_valid && data.format_valid ? 'border-green-500' : 'border-red-500';
resultContainer.innerHTML = `<div class="space-y-1 px-5 py-3 border-2 rounded-md ${borderClasses}">
<div class="flex items-baseline justify-between"><span class="font-medium w-44">Checksum valid:</span><span class="text-right">${data.checksum_valid}</span></div>
<div class="flex items-baseline justify-between"><span class="font-medium w-44">Format valid:</span><span class="text-right">${data.format_valid}</span></div>
<div class="flex items-baseline justify-between"><span class="font-medium w-44">Country Code:</span><span class="text-right">${data.country_code}</span></div>
<div class="flex items-baseline justify-between"><span class="font-medium w-44">VAT Number:</span><span class="text-right">${data.vat_number}</span></div>
<div class="flex items-baseline justify-between"><span class="font-medium w-44">Is registered:</span><span class="text-right">${data.registration_info.is_registered}</span></div>
<div class="flex items-baseline justify-between"><span class="font-medium w-44">Company name:</span><span class="text-right">${data.registration_info.name ?? 'N/A' }</span></div>
<div class="flex items-baseline justify-between"><span class="font-medium w-44">Company address:</span><span class="text-right">${data.registration_info.address ?? 'N/A' }</span></div>
</div>`;
}
});
});
Make sure to replace YOUR-API-KEY with your actual key!
And that’s it! Now we have successfully built a VAT validator in JavaScript!
Never worry about VAT IDs again
VAT validation is a necessary step to collect and submit the correct tax treatment but it can be a hassle to keep track of. However, by automating the VAT validation process, you can save yourself a lot of time and resources.
By building a vat validator with Javascript, you can ensure that your organization is always compliant with the latest VAT regulations. This will save you time and money in the long run, and it will make life much easier for you and your customers.