The replaceWith() method is a powerful tool for replacing DOM child elements with new content. By substituting particular parts with other elements or HTML content, we can quickly change the structure of a web page. All new web browsers support replaceWith().
Syntax:
selector.replaceWith(newContent)
- selector: specifies the element to be replaced
- newContent: specifies the new content or elements that will replace the selected element
Return: No return value.
How the replaceWith() Method Works
The replaceWith() method works by replacing the selected elements with new content or elements. It works on the list of elements targeted by the selector, allowing us to dynamically change the layout or appearance of the page.
Here’s how the replaceWith() method works:
Step 1. Select Elements: We start by selecting one or more elements using a selector. These are the elements to replace.
Step 2. New Content: Next, we specify the new content or elements that will replace the selected elements.
Step 3. Replace Elements: Each chosen element is replaced with the new content or items supplied by the replaceWith(). The new content or elements are inserted in their place when the chosen items are removed from the DOM.
Step 4. DOM Manipulation: After the replacement, the DOM is updated accordingly. Any event handlers or data associated with the replaced elements may be lost unless explicitly transferred to the new elements.
Using replaceWith() modifies the DOM directly, so use it with caution, especially when dealing with event handlers or data associated with the replaced elements.
Replacing Elements with HTML Content Using replaceWith()
To replace elements with HTML content using the replaceWith() method, we can provide the HTML content as a string parameter to the method. Here’s how we can do it:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Replace Content by Clicking Button</title>
</head>
<body>
<div id="content">
This is the old content.
</div>
<button id="replaceBtn">Click Me</button>
<script>
document.getElementById("replaceBtn").addEventListener("click", function () {
var oldContent = document.getElementById("content");
var newContent = document.createElement("div");
newContent.textContent = "This is the new content.";
oldContent.replaceWith(newContent);
});
</script>
</body>
</html>
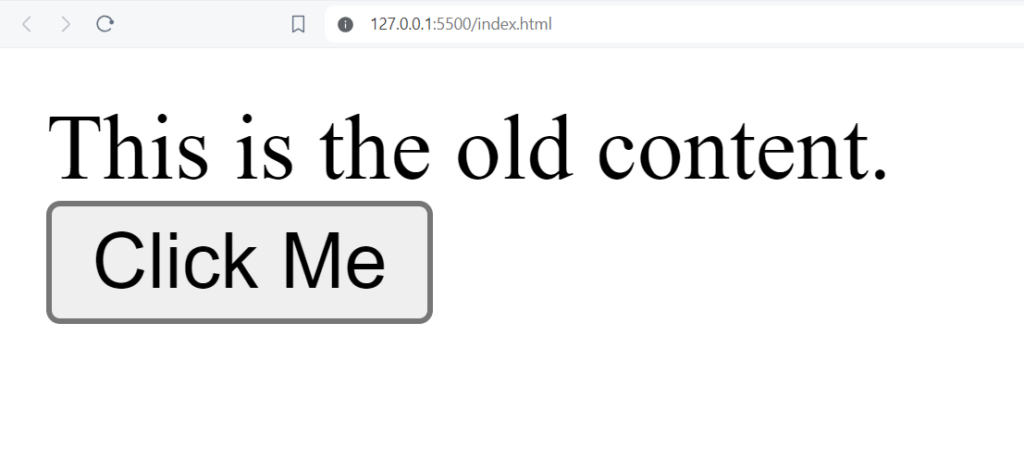
We want to change the original content, “This is the old content” inside a <div> element with the ID “content”. A button element with the ID “replaceBtn” is also present. We want the content to be changed when this button is clicked.
Inside the script: To obtain a reference to the element with the ID “content”, we use document.getElementById(“content”) and used document.createElement(“div”) to create a new <div> element and newContent.textContent to set the text content of the new <div> element to “This is the new content”. Then we replace both content by using the replaceWith() on the old content element.
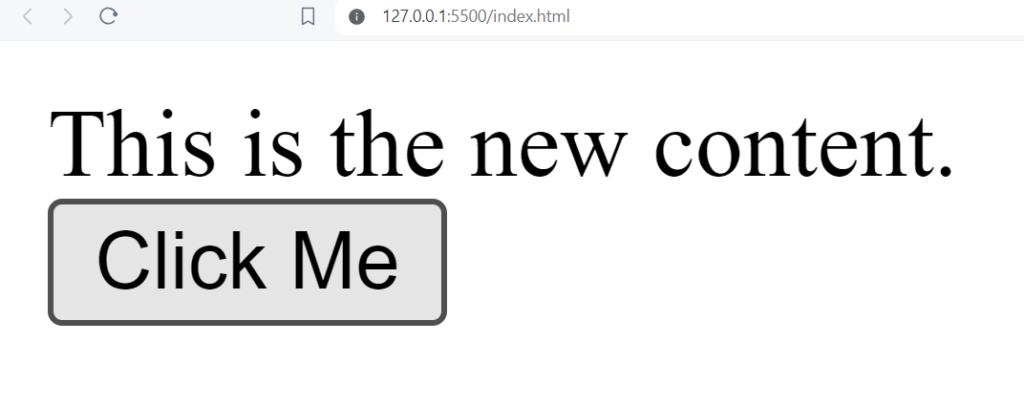
Using HTML DOM replaceWith() with setTimeout for Delayed Element Replacement
With replaceWith() method, we can dynamically replace content within a webpage after a specified timeout using setTimeout().
<!DOCTYPE html>
<html>
<head>
<title>replaceWith() method Example with setTimeout</title>
</head>
<body>
<div id="originalContent">
This is the original content.
</div>
<script>
function replaceContent() {
const originalDiv = document.getElementById("originalContent");
const newParagraph = document.createElement("p");
newParagraph.textContent = "This is the new content.";
originalDiv.replaceWith(newParagraph);
}
setTimeout(replaceContent, 3000);
</script>
</body>
</html>
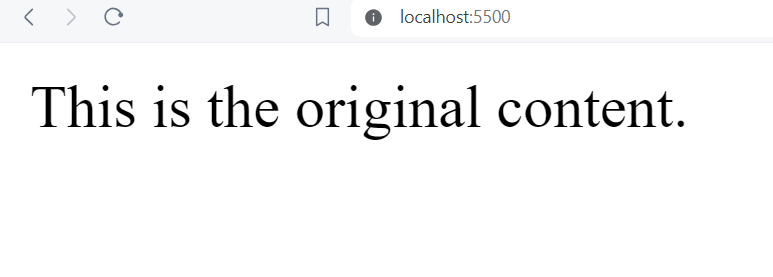
We used setTimeout() function here to delay the execution of the replaceContent() function by 3000 milliseconds, i.e., 3 seconds. This creates a delay before the replacement of the original content occurs.
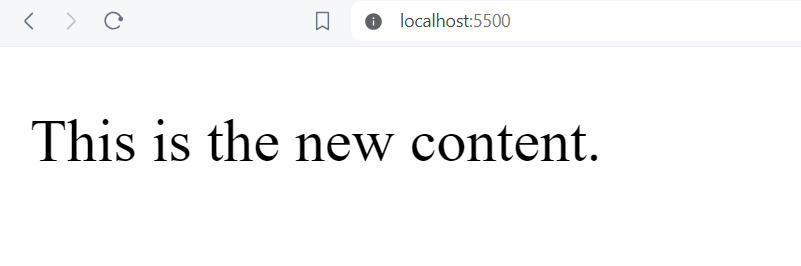
Finding it difficult to understand setTimeout() function? Click here.
Conclusion
In conclusion, replaceWith() method in JavaScript provides an easy way to work with the Document Object Model (DOM). It provides developers with an easy way to dynamically replace components with text or other elements, enabling them to create dynamic and interactive applications.
Also Read: Create a Snake Game Using HTML, CSS, and JavaScript