As a Python developer, you might find yourself lacking in building websites that other developers like JavaScript, PHP, etc. can easily do. Well, you can also build web applications using Python by implementing a super simple framework called Flask.
Flask is a lightweight web framework that can be used in Python to build web applications without the need for any additional programming languages.
One of the best features of Flask is the rendering of dynamic HTML templates, which can be a very quick way to build a website using just a few lines of code. In this article, we are going to find out how we can render HTML templates using Flask in a very beginner way. So let’s get started.
Step 1: Setting Up Environment for Creating Flask Application
- Installing a Code Editor for writing Python code.
- Installing the latest version of Python from the official Python website (https://www.python.org/downloads/)
- Verify Installation by typing the command python –version in the terminal.
- Install Flask by using the command pip install Flask.
- Verify Flask Installation using the command flask –version.
- Create a Project Directory and Open it inside the Code Editor.
Step 2: Create an HTML File for Rendering
We have to create a new folder in the project directory called templates. Create a new file called home.html in the templates folder for writing HTML code which will be rendered.
Below is the HTML Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<h1>Welcome to the HomePage!</h1>
</body>
</html>
Step 3: Writing Code for Render HTML
Now we have to create a Python file app.py where we will be writing code for our Flask app for rending the HTML page we just created.
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def home():
return render_template('home.html')
if __name__ == '__main__':
app.run()
We are importing the render_template function provided by the Flask and then rendering our HTML template in the home route.
Run the app using the command:
python app.py
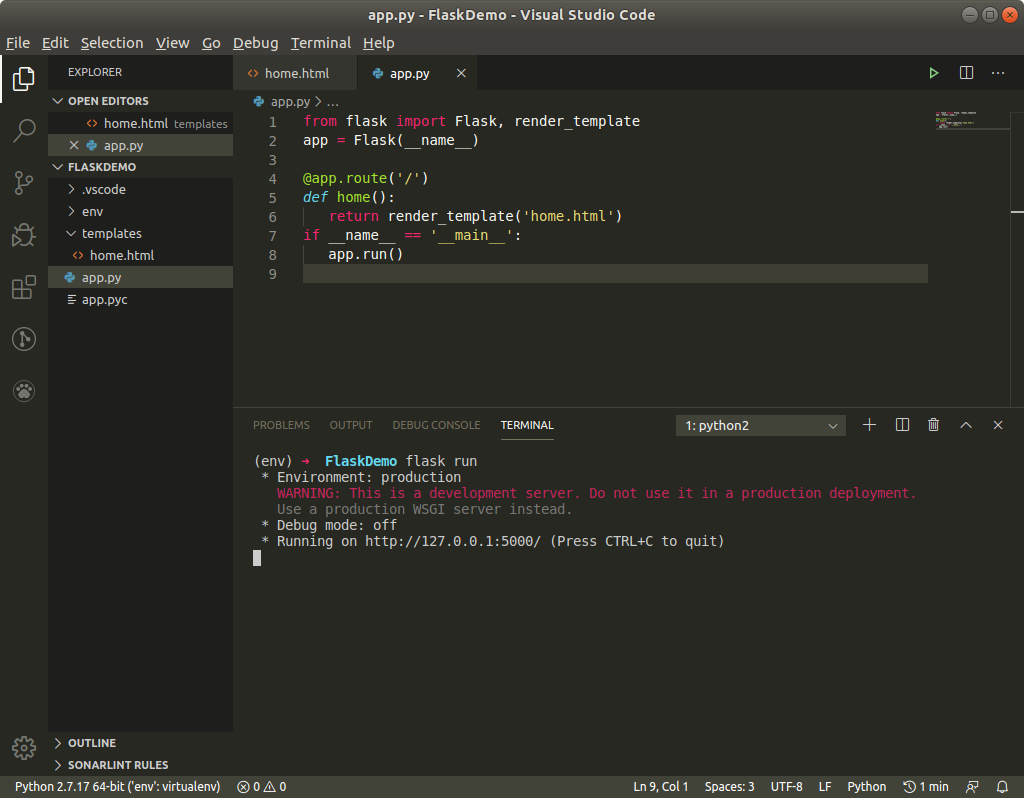
Now, navigate your browser to localhost:5000 to see the output.
Output:
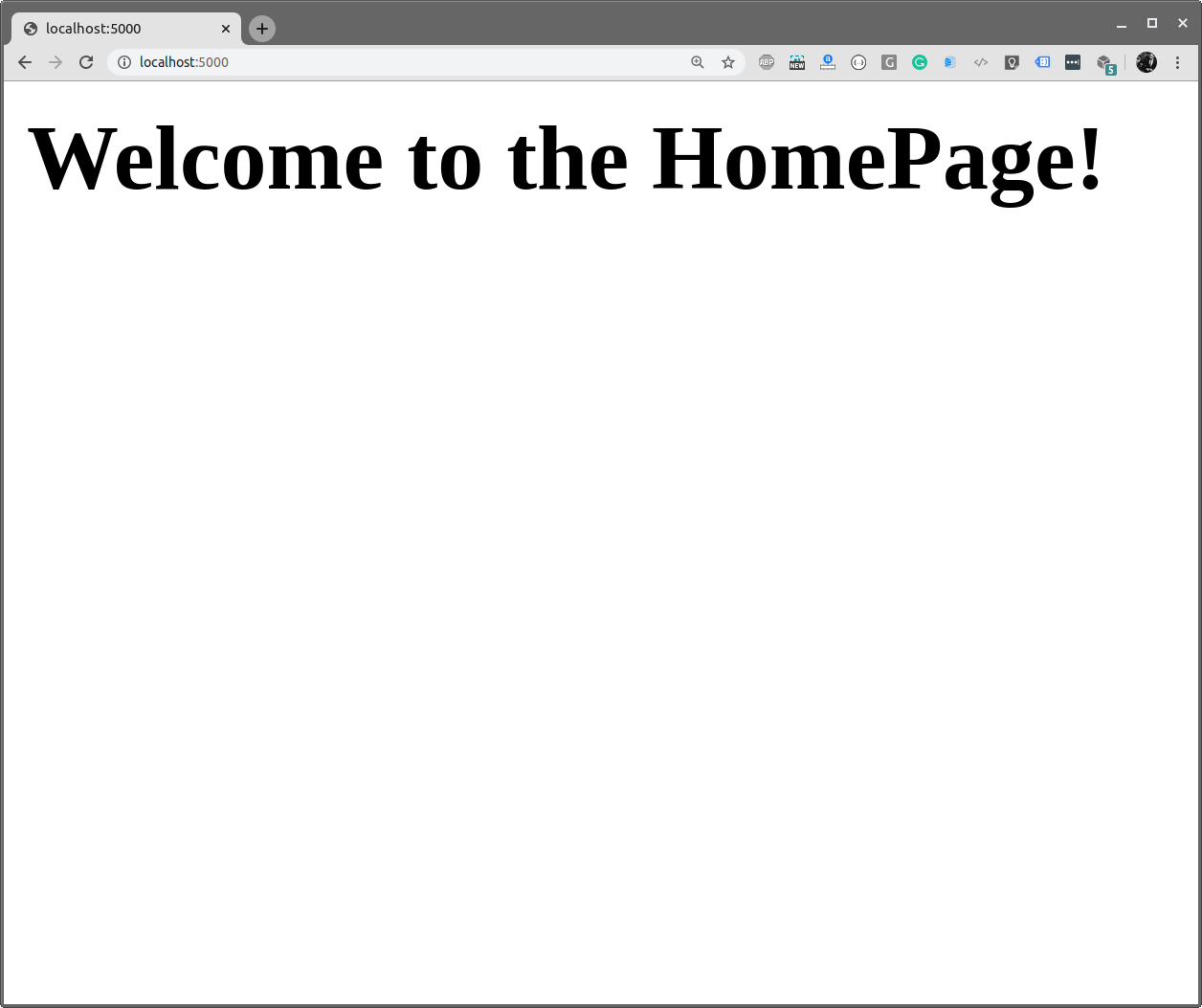
Awesome! You have just rendered an HTML template in the Flask.
Also Read: Getting Started with Python Flask Framework
Frequently Asked Questions (FAQs)
Can Flask render HTML?
Yes, we can use Flask to render HTML pages by importing the render_template function.
How to put HTML code in Python?
To put HTML code in Python, we can either directly include it as strings in our Python code or we can use templating libraries like Jinja for more complex applications. For simplicity, we can use web frameworks like Flask as well.
How do I add multiple HTML files to Flask?
In Flask, create a folder named “templates” and put all HTML files in that folder. Then you can use render_template to load and render these files accordingly.
What is render_template in Flask?
The render_template is a Flask function that we can import to load HTML templates from the “templates” folder and render them with the required data to create dynamic web pages.
How to connect HTML and CSS in Flask?
You must first put the HTML file in the “templates” folder and the CSS file in the “static” folder, then in the head section of the HTML file you have to insert the code <link rel=’styleSheet” href='{{ url_for(‘static)’, filename=’style.css’) } }”>.
Summary
In their tutorial, we learned how to render a basic HTML page using Flask in Python. Flask is not limited to small projects. You can also use Flask web applications for projects with advanced functionality like taking user inputs, giving calculated outputs, and we can even build fully-fledged and complex web applications. To deep dive into Flalsk and learn more about it click here.