CSV (Comma Separated Values) is a plain text file format used to store tabular data, commonly in spreadsheets. In a CSV file, every line represents a record and each field in that line of record is separated using a comma. We mainly use CSV to import and export data between applications. Python provides us with an easy way of CSV file handling using the CSV module. In this article, we’ll explore the various ways of reading and writing into CSV files, along with some comprehensive examples.
Working with CSV Files in Python
Python allows us to easily perform various operations on CSV files using the CSV module.
The two operations we’ll look at in this article are as follows:
- Reading CSV files
- Writing to CSV files
Before we can start working with CSV files, we must always make sure that we have imported the CSV module into our file as follows:
import csv
Now let’s understand the above operations in detail one by one.
1. Reading a CSV file
To read a CSV file we use the reader() method provided in the CSV module. This returns a reader object which we can use to access the records in the file. However, to read a file, we first need to open the file using the open() method. The open() method takes two parameters – the name of the file to be opened and the mode in which it has to be opened. Since we are reading the file, we open the file in read mode by setting mode=’r’. Let us understand this better with an example.
Let us consider a file marks.csv containing the following data:
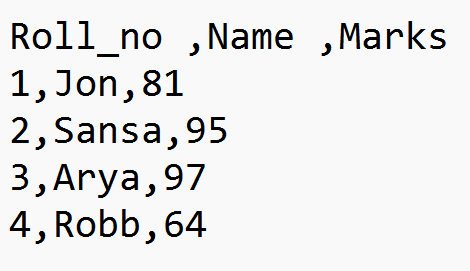
We want to read each record (each line) from the file and display it. This can be done as follows:
import csv
with open('marks.csv', mode='r') as file:
reader = csv.reader(file)
for row in reader:
print(row)
Here, we first import csv in our file. Then we open our file marks.csv as a file in read mode. The open() method returns a file object which we can pass into the reader() method. The reader() method reads the CSV file and returns a reader object which we can iterate over to obtain the records from the file. The reader object is stored in the reader variable. We then run a for loop through the reader object and print each row of the file.
Output:
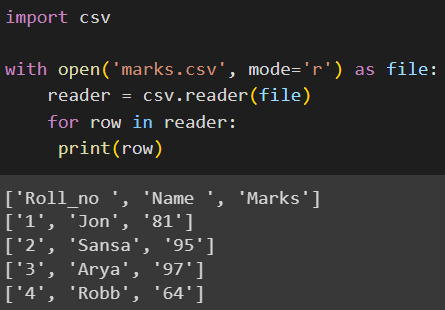
Keep in mind that the marks.csv must be in the same directory as the Python file or else, we must specify the path to the directory in which the file is present. Also, in order to read a file, it must already exist in the specified path, or else an error is thrown.
2. Writing to CSV Files
Similar to the reader() function, CSV provides a writer() function that helps us write data into a csv file by creating a writer object. This object converts the data entered into a delimiter string in order to store it in our csv file, but first, we need to open our file using open(). We pass the name of the file and mode=’w’ to open() to indicate that we’re opening the file in write mode.
There are two methods by which we can write into a file using csv.writer(), these are as follows:
- writerow(): This method writes a single row of data at a time to the CSV file. It takes either a list. tuple or string as its input.
- writerows(): This method is used to write multiple rows of data at a time into a CSV file. It takes in a list of rows as its input.
Now we will look at some examples of how we can write data into a file using these two methods.
2.1 Writing to CSV files using writerow()
Consider a file marks.csv:
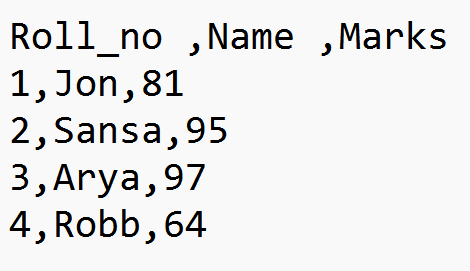
We want to write two new rows into this file using writerow(). This can be done as follows:
import csv
row1 = [5,'Bran',22]
row2 = [6, 'Ned',49]
with open('marks.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerow(row1)
writer.writerow(row2)
Firstly, we import csv into our file. Then we declare two lists row1 and row2 which store the records that will be written into marks.csv. Then we open marks.csv in write mode (‘w’) as a file. Then we initialize the writer object using writer() and pass our file into the writer() method. This returns a writer object. Then we use writerow() on the writer object to write our rows one by one into marks.csv.
Output:
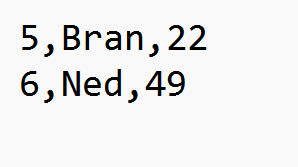
Here we notice that the old data has been overwritten while writing to a file. It is also crucial to note that while working with files in write mode if the file doesn’t exist, it is automatically created in the current directory.
2.2 Writing to CSV files using writerows()
Let us consider the same file marks.csv as the previous example.
Before writing to the file, the file contents are as follows:
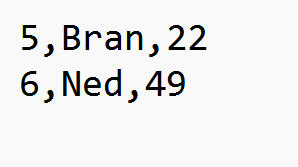
We want to write a list of 3 new rows into marks.csv. This can be done as follows:
import csv
rows = [[7,'Jamie',78], [8, 'Cersei',91], [10, 'Tyrion',88]]
with open('marks.csv', mode='w', newline='') as file:
writer = csv.writer(file)
writer.writerows(rows)
We first import csv and then declare a list called rows, which contains a list of 3 records to be written into marks.csv. To write into the file, we open it using the open() function in write mode (‘w’). Then we initialize the writer object using the writer() method. Finally, we use writerows() on the writer object to write all 3 records at a time into marks.csv.
Output:
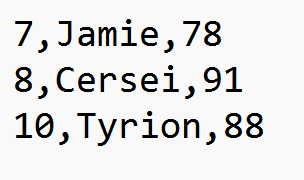
Just like the previous example, since we have written into the file, the previous data in the file has been overwritten. To prevent data from being overwritten, we can set mode=’a’ while opening the file, which opens the file in append mode. Hence, the new data is only appended and doesn’t overwrite data already in the file.
Conclusion
The CSV module in Python offers a flexible way to read and write CSV files by providing functions like reader(), writer() and so on. In this article, we’ve seen in detail how we can use the CSV module to read files using the reader object and how to write to files using the writer object. Within the writer object, we have also looked at two functions – writerow() and writerows() which help us write data into files. Mastering CSV file handling can be very handy in various fields that deal with records.
Reference
https://stackoverflow.com/questions/41585078/how-do-i-read-and-write-csv-files