Amongst the many libraries in Python, numpy is the one feature rich in all sorts of arithmetic and numerical functions. This article sets out to explain the execution of one of the fundamental arithmetic operations – subtraction using the numpy library in Python. For starters, this might seem like the work of a calculator, but with tons of data that is to be handled in machine learning, you might need to think otherwise.
Following are the different techniques to perform subtraction. All these shall be done with the aid of the subtract() function called from the numpy library.
- Scalars subtraction
- Arrays subtraction
- Subtracting array & scalar
- Subtracting arrays of dissimilar sizes
- Selective subtraction
We will look at all these examples in this article, but first, let’s understand the syntax of the numpy.subtract() function.
Syntax of numpy.subtract()
Let us get started with the basic components of the subtract( ) function as shown below.
Syntax:
numpy.subtract(x1, x2, where=True, dtype=None)
Parameters:
- x1, x2 – scalars or N-dimensional arrays to be subtracted
- where – the location within the array that is only to be subtracted excluding others
- dtype – the data type of the entities that are to be subtracted
Now that we have gone past the syntax, let us import the numpy library & try out each of the subtraction techniques.
Import Syntax:
import numpy as np
Examples of numpy.subtract()
Let’s now look at the techniques we mentioned in the beginning using the numpy.subtract() function.
Scalars Subtraction
Scalars are numerical entities that unlike arrays are limited only to possess a single number. Given below is a simple code to subtract scalars in Python.
Example:
x1 = 89
x2 = 47
np.subtract(x1, x2)
Output:
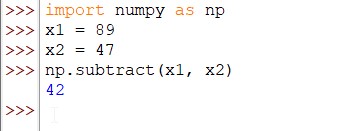
It is to be noted that the first element specified within the subtract( ) function which in this case is ‘x1’ is the element that is being subtracted & the succeeding element, x2 is the one that is being removed from ‘x1’. This holds good for subtracting arrays too!
Arrays Subtraction
Arrays have multiple numerals nested within them. Let us see how the subtract( ) function can be used to determine the difference between 1-dimensional arrays.
Example:
ar1 = np.array([0, 9, 8])
ar2 = np.array([1, 3, 5])
np.subtract(ar1, ar2)
Output:
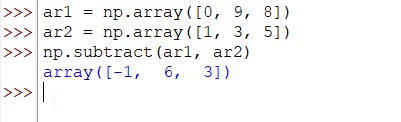
Subtracting Array & Scalar
Python offers us the provision to subtract two dissimilar entities – a single number (i.e.) scalar from a bunch of numbers (i.e.) arrays using the subtract( ) function. This is made possible by removing the input scalar from each numerical entity listed within the 2-dimensional array as demonstrated below.
Example:
ar3 = np.array([[4, 8, 7], [2, 9, 4]])
np.subtract(ar3, 5)
Output:
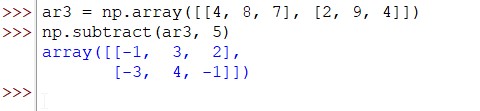
Subtracting Arrays of Dissimilar Sizes
Though it is a no-brainer to find the difference between arrays of the same size, there might be instances when one needs to subtract 2 arrays that are of different sizes. But Python allows such flexibility with a stipulation that both arrays must have a similar count of columns.
Let us have a look at the following example which details on this special case subtraction using a 1-dimensional and a 2-dimensional array.
Example:
ar1 = np.array([0, 9, 8])
ar3 = np.array([[4, 8, 7], [2, 9, 4]])
np.subtract(ar3, ar1)
Output:
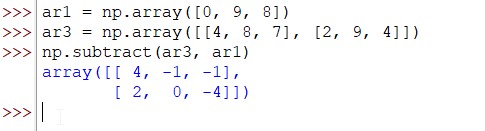
Selective Subtraction
Using this feature one can specify the places in which the subtraction ought to be carried out such that the other entities in the array remain undisturbed. In order to make this happen, the where component as stated in the syntax earlier shall be summouned as shown in the below code.
Example:
ar3 = np.array([[4, 8, 7], [2, 9, 4]])
ar4 = np.array([[4, 7, 1], [7, 2, 7]])
ar5 = np.subtract(ar3, ar4, where=[True, False, False])
print(ar5)
Output:
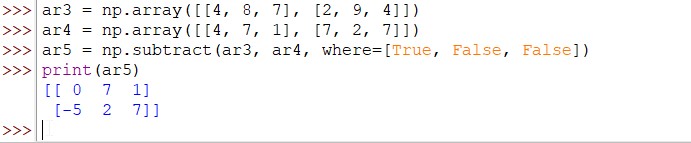
It can be seen from the above image that only the first elements in each row of the arrays ar3 and ar4 are subjected to subtraction, while the rest of the elements in the resultant array are taken directly from the ‘ar4’ which is the array used for subtraction.
Conclusion
Now that we have reached the end of this article, hope it has elaborated on how to subtract using the subtract( ) function in Python programming. Here’s another article that can be your definitive guide to indentation in Python. There are numerous other enjoyable and equally informative articles in CodeforGeek that might be of great help for you to gain valuable insights. Until then, ciao!