It is time for us to delve into the vast library of Python packed with numerical functions – the numpy library and this time, we will explore a rather strange function. It is very strange that at first sight, one might mistake it to be an abomination within Python.
The function of interest would be the numpy.positive( ) function which returns the element-wise numerical positives for any given input array.
That might seem a bit no-brainer, but there is a silver lining! This function has nothing to do with the conversion of negative numbers in the input array into their positive equivalents. So, what exactly, is it destined to do? Let us have a closer look to find it out through each of the following sections:
- Syntax of positive( ) Function
- Use Cases of the numpy.positive( )
- numpy.positive( ) on N-Dimensional Arrays
As always, let us get things started by importing the numpy library using the following code:
import numpy as np
Syntax of positive( ) Function
Given below is the syntax of the positive( ) function containing all the basic constituents required for its effective functioning.
Syntax:
numpy.positive(x, out=None, *, where=True, dtype=None)
where,
- x – Input scalar or N-dimensional array whose numerical positives are to be returned
- out – an optional construct set to none by default and could be used to store the results in the desired array which is similar in size to that of the output
- * – kwargs or keyword argument is an optional construct that is used to pass keyword variable length of argument to a function
- where – an optional construct which is used to apply the function only at the specified positions when set to True (default setting) or skip the positions when set to False
- dtype – an optional construct used to specify the data type that is to be returned
Use Cases of the numpy.positive( )
In this section, we shall construct an array & try running it through the positive( ) function to have a look at the outcome.
ar1 = np.array([[-8, 4, 2, -9],
[-5, 0, 2, -3]])
r1 = np.positive (ar1)
print (r1)
Given below are the results for the above code:
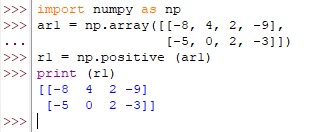
One might think that nothing has happened & the function has just returned whatever has been fed as its input. Let’s have a look from a different perspective to really get to know what has happened.
ad1 = print (id(ar1))
ad2 = print (id(r1))
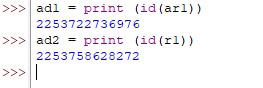
Now that the results are stored in a different location, one can argue further that this might as well be done by using a copy( ) function. But the positive( ) function offers flexibility to bend the data type which does not happen in the case of the copy( ) function.
r2 = np.positive (ar1, dtype = float)
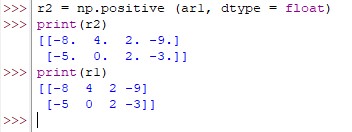
This function works well with every data type except for Boolean which might return the following error.
UFuncNoLoopError: ufunc 'positive' did not contain a loop with signature matching types
numpy.positive( ) on N-Dimensional Arrays
Let us now apply the positive( ) function to an N-dimensional array, but this time we shall store its result in another array that is to be created to be of the same size.
ar1 = np.array([[-8, 4, 2, -9],
[-5, 0, 2, -3]])
r2 = np.zeros((2,4))
print(r2)
np.positive (ar1, out=r2, dtype=float)
print(r2)
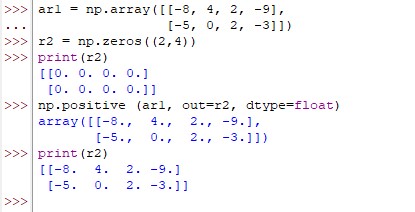
Conclusion
Now that we have reached the end of this article, hope it has elaborated on how to use the positive( ) function from the numpy library with some use cases. Here is another article that details and emphasizes the importance of using indentations in Python programming. There are numerous other enjoyable and equally informative articles in CodeforGeek that might be of great help to those who are looking to level up in Python.
Reference
https://numpy.org/doc/stable/reference/generated/numpy.positive.html