NodeJs V8 is a built-in module used to get various information like heap memory, it exposes APIs that are specific to the version of V8. Since NodeJS itself build on the V8 engine it is important to know about these details.
V8 is a JavaScript engine developed under the chromium project by Google. It was created for Chromium-based browsers such as Google Chrome, but due to its fast execution speed and open-source ability later it was utilized to create many other technologies, one of them being Node.js, MongoDB, etc. V8 can execute JavaScript code directly without generating the intermediate code.
The memory limit of V8
The major problem with the V8 engine is limited memory, for a 32-bit system it is 512MB RAM which can be increased up to 1GB, and for 64-bit it is 1GB up to 1.7GB. There is a need to manage memory, for running under the memory limit.
To manage memory it is important to have information about the heap memory, total heap size, used heap size, total physical memory, total available size, etc. To get this information NodeJS V8 provides various methods that can be used by importing the V8 module.
NodeJs V8 Module
V8 module is a built-in module in NodeJS that provide various methods used to get different types of information regarding the heap. For using its method, it is required to import it using the below syntax.
Syntax:
const v8 = require('v8');
Methods of the V8 Module
Let’s look at the methods available within the NodeJS V8 Module.
1. getHeapStatistics()
This method returns much information about the heap memory such as total heap size, total heap size executable, total physical memory, total available size, used heap size, and heap size limit.
Syntax:
v8.getHeapStatistics()
Example:
const v8 = require('v8');
const result = v8.getHeapStatistics();
console.log(result);
Output:
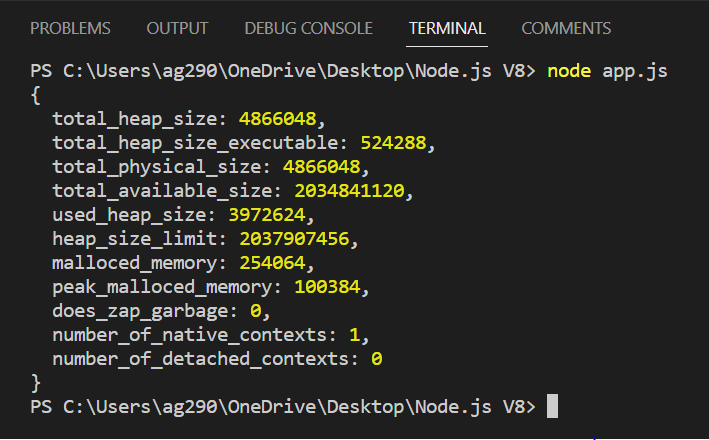
2. getHeapSpaceStatistics()
This method returns an array containing 5 objects, new space, old space, code space, map space, and large object space.
Syntax:
v8.getHeapSpaceStatistics()
Example:
const v8 = require('v8');
const result = v8.getHeapSpaceStatistics();
console.log(result);
Output:
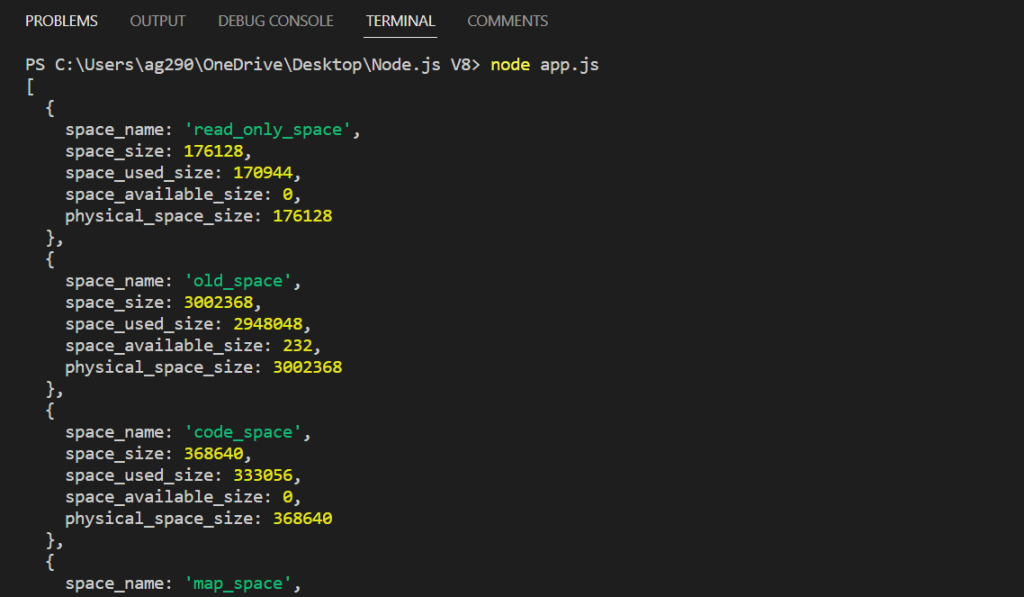
3. getHeapSnapshot()
This method takes a snapshot of the current V8 heap and returns a readable stream.
Syntax:
v8.getHeapSnapshot()
Example:
const v8 = require('v8');
const result = v8.getHeapSnapshot();
console.log(result);
Output:
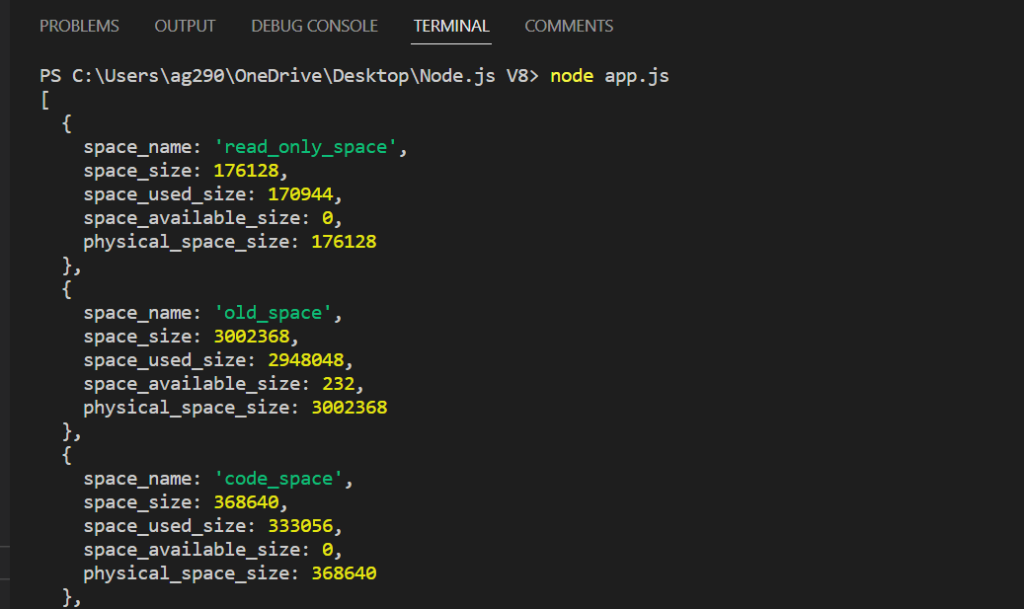
4. getHeapCodeStatistics()
This method returns an object containing the statistics about the code and its metadata in the heap.
Syntax:
v8.getHeapCodeStatistics()
Example:
const v8 = require('v8');
const result = v8.getHeapCodeStatistics();
console.log(result);
Output:
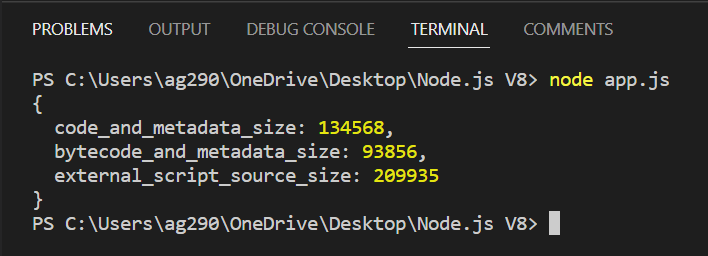
5. writeHeapSnapshot()
This method takes a file path as an argument and generates the snapshot of the current V8 heap and writes it to the JSON on the given file path and returns the filename where the snapshot is saved.
Syntax:
v8.writeHeapSnapshot(filePath)
where the filePath is the path of the file where the snapshot gets stored.
Example:
const v8 = require('v8');
const result = v8.writeHeapSnapshot("./heapdump.heapsnapshot");
console.log(result);
Output:
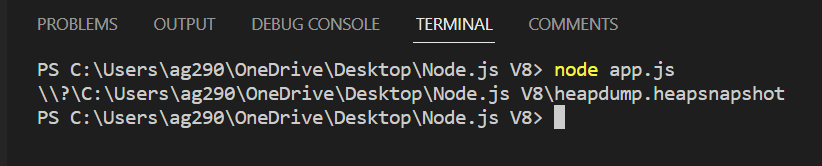
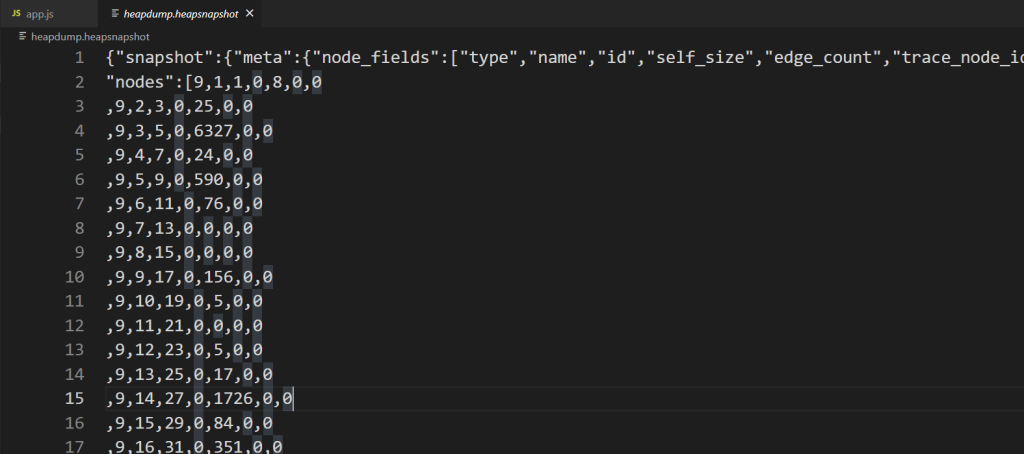
Serialization API
V8 module provides methods used to serialize and deserialize the values. This makes the JavaScript values compatible for execution, serialize the values into Buffer, and then deserialize them to the original state.
serialize()
This method takes JavaScript values, serializes them, and returns a buffer.
Syntax:
v8.serialize(value)
where value is a JavaScript value we want to serialize.
Example:
const v8 = require('v8');
const result = v8.serialize("Hello World!");
console.log(result);
Output:
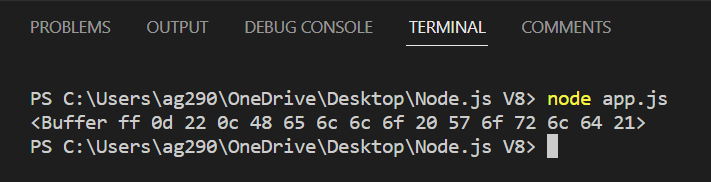
deserialize()
This method takes buffers, deserializes them, and returns the original text. It reverses the operation of serialize() method.
Syntax:
v8.deserialize(buffer)
where the buffer is a buffer we want to deserialize.
Example:
const v8 = require('v8');
let result = v8.serialize("Hello World!");
result = v8.deserialize(result);
console.log(result);
Output:
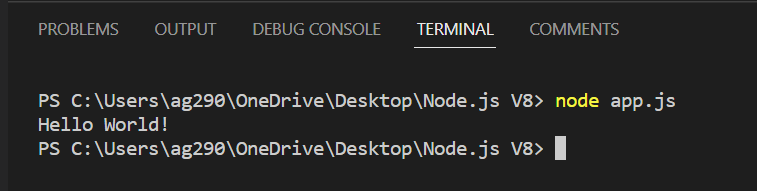
Summary
NodeJS V8 module provides various methods used to get the different information about the heap and physical memory in order to manage the processes so that the V8 engine doesn’t run out of memory since V8 has limited memory. We hope this tutorial helps you to understand NodeJS V8.
Reference
https://nodejs.org/api/v8.html