At this point, we have covered a lot of topics in our Next.js series that are enough to help you build your own very first Next.js app. But wait! Once you have built your next.js app and hosted it, you want to get a lot of views. To get views, your application must be highly optimized and can be rendered pages easily to provide a better user experience and also for SEO, so let’s see how we can achieve that.
Follow our Next.js series: CFG Next.js Tutorials
8 Ways to Optimize Your Next.js App
1. Next.js Image Component
If you are using a regular HTML <img> tag for inserting images then the first thing you can do to optimise the website is replace it with the Next.js <Image> component.
This component will automatically handle image optimization by using formats like WebP and resizing images based on device screen size. It also loads offscreen images slowly (lazy loading) as they are not directly visible on the screen. This reduces the initial page load time and improves SEO by loading images faster for user experience.
import Image from 'next/image';
<Image
src="/image.jpg"
alt="Description"
width={500}
height={300}
/>
2. Dynamic Imports
Sometimes we import a lot of modules and some of them are used only sometimes. For example, let’s say we have imported a module “x” which is used only when a user tries to open the register page, but by default, the application imports it even if the user does not open the page. This makes the overall bundle size unnecessarily increase and slows down the initial loading speed.
To solve this problem, we can import a module dynamically. So until a module is needed, it is not being imported, so the initial loading speed is high.
In this way, we can increase the overall performance of the application to the next level by lazy loading the modules that do not need to be loaded initially.
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('../components/DynamicComponent'));
3. Specific Imports
To optimize the bundle size, we can import only a specific function or component that we need instead of importing the entire module.
import { specificFunction } from 'module';
And that’s it. This small change can reduce a lot of space.
4. Lazy Load Images
Similarly, we can also lazy load images. Images that do not need to be loaded initially, like the images at the bottom of a page only need to be loaded when the user scrolls near them. So these types of offscreen images can be lazy loaded using the attribute loading=”lazy” in the Next.js <Image> component so that without decreasing the image size and quality we can provide a faster browsing experience to our users.
<Image
src="/image.jpg"
alt="Description"
width={500}
height={300}
loading="lazy"
/>
5. Optimize Font Loading
Another small change that can increase the performance is self-hosting fonts or using the ‘font-display: swap’ CSS property.
Self-hosting fonts reduce dependence on external sources and provide faster rendering of text.
And using ‘font-display: swap’ allows browsers to use system fonts as placeholders until our custom fonts are loaded to avoid render-blocking.
@font-face {
font-family: 'CustomFont';
src: url('/fonts/custom-font.woff2') format('woff2');
font-display: swap;
}
6. Optimizing Links
In Next.js we use the <Link> component from next/link to link to different pages. It loads the assets of the linked page by default. To prevent this we can set the ‘prefetch’ prop to ‘false’ and then prefetching will only happen when a user hovers over the link.
import Link from 'next/link';
<Link href="/about" prefetch={false}>
<a>About</a>
</Link>
7. Remove Unused Packages
There are many modules and packages that you have installed but not used and they are just increasing the overall size of the application. So make sure to remove them to reduce the bundle size.
You can check all the installed packages in package.json or use a tool like Depcheck to check the packages that are no longer used and can be removed without any worries.
npm uninstall unused-package
8. Use Bundle Analyzer
We can use the bundle analyzer from @next/bundle-analyzer to visually analyze our application bundles and take action accordingly. Let’s see how to use it.
Run the below command to install the bundle analyzer:
npm install @next/bundle-analyzer
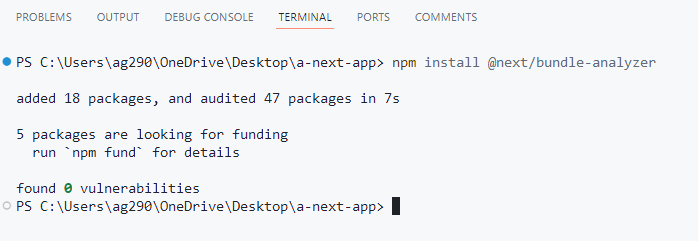
Now just execute the below command to analyse:
set ANALYZE=true
npm run build
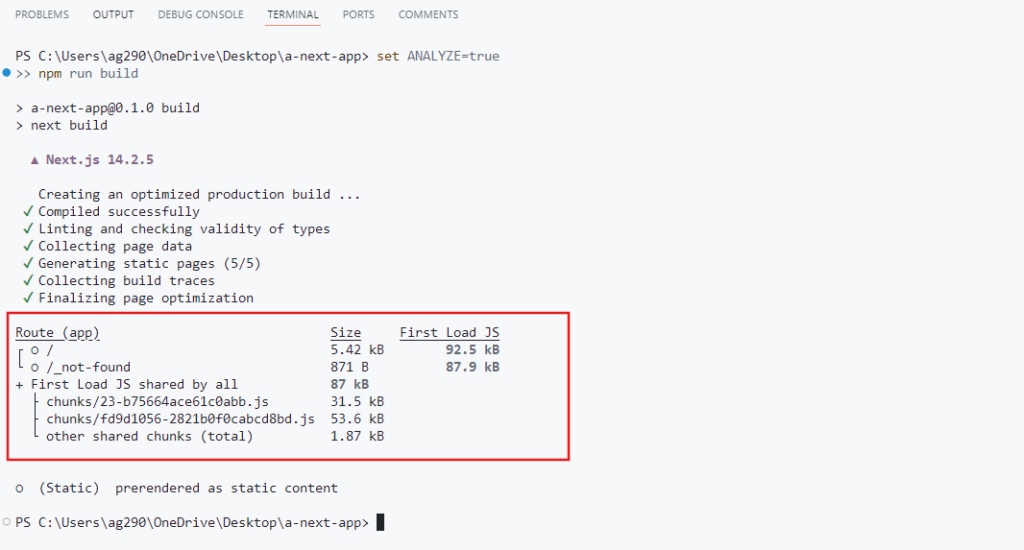
Now you can identify large modules, replace them, implement lazy loading, remove unused modules, and optimize imports.
Summary
In short, optimizing Next.js is easy and important for better performance. In this article, we have explained the 8 best ways and if you want to achieve the peak of optimization, then you know that you can export your Next.js generated HTML/CSS/JS as a static file and host it on a static server for faster rendering. This is what we will learn to do in our next tutorial.
Reference
https://nextjs.org/docs/pages/building-your-application/optimizing